Changelog #37
·
Wait for Event
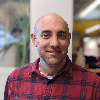
CTO, Trigger.dev
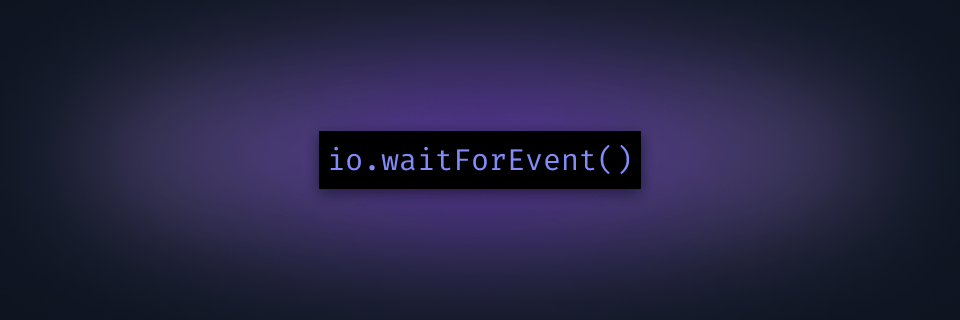
Up until now, you could only trigger a new job run when sending an event to Trigger.dev using eventTrigger()
:
_17client.defineJob({_17 id: "payment-accepted",_17 name: "Payment Accepted",_17 version: "1.0.0",_17 trigger: eventTrigger({_17 name: "payment.accepted",_17 schema: z.object({_17 id: z.string(),_17 amount: z.number(),_17 currency: z.string(),_17 userId: z.string(),_17 }),_17 }),_17 run: async (payload, io, ctx) => {_17 // Do something when a payment is accepted_17 },_17});
Now with io.waitForEvent()
, you can wait for an event to be sent in the middle of a job run:
_12const event = await io.waitForEvent("🤑", {_12 name: "payment.accepted",_12 schema: z.object({_12 id: z.string(),_12 amount: z.number(),_12 currency: z.string(),_12 userId: z.string(),_12 }),_12 filter: {_12 userId: ["user_1234"], // only wait for events from this specific user_12 },_12});
By default, io.waitForEvent()
will wait for 1 hour for an event to be sent. If no event is sent within that time, it will throw an error. You can customize the timeout by passing a second argument:
_18const event = await io.waitForEvent(_18 "🤑",_18 {_18 name: "payment.accepted",_18 schema: z.object({_18 id: z.string(),_18 amount: z.number(),_18 currency: z.string(),_18 userId: z.string(),_18 }),_18 filter: {_18 userId: ["user_1234"], // only wait for events from this specific user_18 },_18 },_18 {_18 timeoutInSeconds: 60 * 60 * 24 * 7, // wait for 1 week_18 }_18);
This will allow you to build more complex workflows that simply were not possible before, or were at least a pain to implement. We're excited to see what you build with this new feature!
Read more about it in the docs.
How to update
The trigger.dev/*
packages are now at v2.2.6
. You can update using the following command:
_10npx @trigger.dev/cli@latest update