Update parent & root metadata
You can now update the metadata of parent and root runs from child runs.
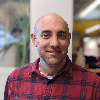
CTO, Trigger.dev
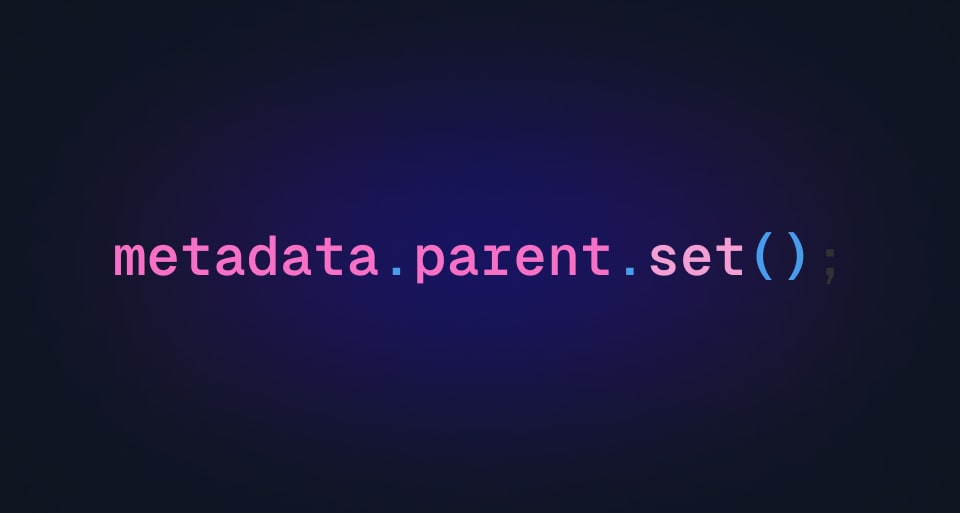
Overview
Starting in 3.3.9, runs that have been triggered by a parent task will now be able to mutate the parent runs's metadata:
import { task, metadata } from "@trigger.dev/sdk/v3";export const myParentTask = task({ id: "my-parent-task", run: async (payload: { message: string }) => { // Do some work metadata.set("progress", 0.1); // Trigger a child task await childTask.triggerAndWait({ message: "hello world" }); },});export const childTask = task({ id: "child-task", run: async (payload: { message: string }) => { // This will update the parent task's metadata to set the progress to 0.5 metadata.parent.set("progress", 0.5); },});
In addition to metadata.parent
, you can also mutate the metadata.root
, which is the highest level parent task in the task tree. This is useful when you have a task that triggers another task, which triggers another task, and so on. The metadata.root
will always point to the top-level parent task.
import { task, metadata } from "@trigger.dev/sdk/v3";export const myParentTask = task({ id: "my-parent-task", run: async (payload: { message: string }) => { // Do some work metadata.set("progress", 0.1); // Trigger a child task await childTask.triggerAndWait({ message: "hello world" }); },});export const childTask = task({ id: "child-task", run: async (payload: { message: string }) => { // In this case, metadata.root and metadata.parent are the same metadata.root.set("progress", 0.5); await grandChildTask.triggerAndWait({ message: "hello world" }); },});export const grandChildTask = task({ id: "grand-child-task", run: async (payload: { message: string }) => { // This will update the root task's metadata to set the progress to 0.9 metadata.root.set("progress", 0.9); },});
All of the update methods are available on metadata.parent
and metadata.root
:
metadata.parent.set("progress", 0.5);metadata.parent.append("logs", "Step 1 complete");metadata.parent.remove("logs", "Step 1 complete");metadata.parent.increment("progress", 0.4);metadata.parent.decrement("otherProgress", 0.1);metadata.parent.stream("llm", readableStream);metadata.root.set("progress", 0.5);metadata.root.append("logs", "Step 1 complete");metadata.root.remove("logs", "Step 1 complete");metadata.root.increment("progress", 0.4);metadata.root.decrement("otherProgress", 0.1);metadata.root.stream("llm", readableStream);
You can also chain the update methods together:
metadata.parent .set("progress", 0.1) .append("logs", "Step 1 complete") .increment("progress", 0.4) .decrement("otherProgress", 0.1);
Why is this useful?
This feature is useful when you have a parent task that triggers child tasks and you want to update the parent task's metadata based on the child task's progress. This allows you to have a single source of truth for the progress of the entire task tree. Combined with our Realtime API, you can build powerful dashboards that show the progress of all tasks in a task tree in real-time.
Example
We've built an example "CSV importer" full-stack app that triggers a child task for each row in the CSV file. The child task updates the parent task's metadata with the progress of the import:
You can find the full example in our examples repository
Next steps
Checkout our Metadata docs to learn more about how you can use metadata in your tasks. If you have any questions or need help, feel free to reach out to us on our Discord channel or message us on X/Twitter.