Changelog #67
Improved typesafe task triggering
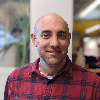
CTO, Trigger.dev
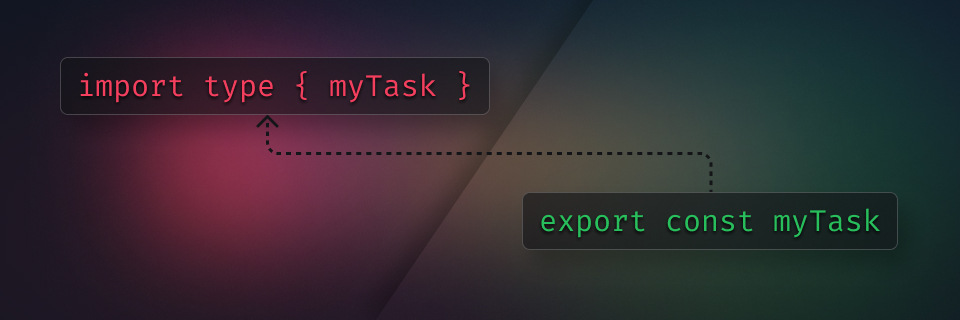
Currently the only way to trigger a task in a typesafe way is to use the Task.trigger
instance method:
This is great for most cases, but importing the task means importing anything the task depends on. This can lead to bloated applications, or worse, build failures because of some esoteric dependency that doesn't work when included in a Next.js 14.2.4 project (for example).
As of v3.0.0-beta.41
, you can now trigger tasks using the tasks.trigger
SDK function, passing in the type of the task only as a generic parameter:
By importing only the type of the task, any dependencies the task has are not included in the build, and you get full type checking on the task payload, ID, and output.
This works with batchTrigger
as well:
In addition, the handle
returned from trigger
and batchTrigger
now carries extra type information about the output of the task, which can be used to retrieve the run output in a typesafe way:
_15import { tasks, runs } from "@trigger.dev/sdk/v3";_15import type { emailSequence } from "~/trigger/emails";_15// 👆 **type-only** import_15_15async function main(email: string, name: string) {_15 // Pass the task type to `trigger()` as a generic argument, giving you full type checking_15 const handle = await tasks.trigger<typeof emailSequence>("email-sequence", {_15 to: email,_15 name,_15 });_15_15 const run = await runs.retrieve(handle);_15_15 return run.output; // 👈 run.output is now typed_15}
There's more! We included a polling helper function to the runs
SDK module, which can be used to poll for the completion of a task run:
_16import { tasks, runs } from "@trigger.dev/sdk/v3";_16import type { emailSequence } from "~/trigger/emails";_16// 👆 **type-only** import_16_16async function main(email: string, name: string) {_16 // Pass the task type to `trigger()` as a generic argument, giving you full type checking_16 const handle = await tasks.trigger<typeof emailSequence>("email-sequence", {_16 to: email,_16 name,_16 });_16_16 // Poll for the completion of the task run_16 const run = await runs.poll(handle, { pollIntervalMs: 1000 });_16_16 return run.output; // 👈 run.output is now typed and complete_16}
We've also added a triggerAndPoll
helper function to the tasks
SDK module, which can be used to trigger a task and poll for the completion of the task run in one go:
_18import { tasks } from "@trigger.dev/sdk/v3";_18import type { emailSequence } from "~/trigger/emails";_18_18async function main(email: string, name: string) {_18 // Pass the task type to `triggerAndPoll()` as a generic argument, giving you full type checking_18 const run = await tasks.triggerAndPoll<typeof emailSequence>(_18 "email-sequence",_18 {_18 to: email,_18 name,_18 },_18 {_18 pollIntervalMs: 1000,_18 }_18 );_18_18 return run.output; // 👈 run.output is now typed and complete_18}
NOTE
These new SDK functions are meant to be used from your server-side code only.
They also aren't meant to replace Task.triggerAndWait
or Task.batchTriggerAndWait
when running inside another task.
You'll find these updates in the @trigger.dev/[email protected]
package. Give it a try and let us know what you think, or if we've missed anything!