Changelog #68
Task delays and TTLs
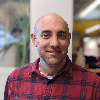
CTO, Trigger.dev
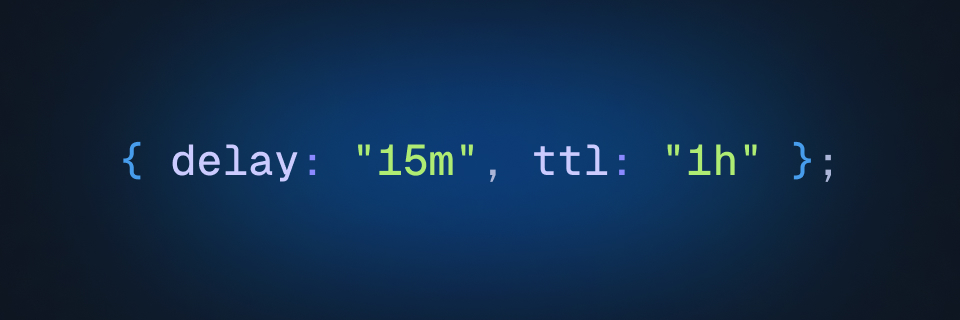
You can now pass two additional options when triggering a task: delay
and ttl
.
Delays
When you want to delay the start of a task, you can now pass a delay
option when triggering or batch triggering a task. The delay
option accepts a number of different formats:
_13// Delay the task run by 1 hour_13await myTask.trigger({ some: "data" }, { delay: "1h" });_13// Delay the task run by 88 seconds_13await myTask.trigger({ some: "data" }, { delay: "88s" });_13// Delay the task run by 1 hour and 52 minutes and 18 seconds_13await myTask.trigger({ some: "data" }, { delay: "1h52m18s" });_13// Delay until a specific time_13await myTask.trigger({ some: "data" }, { delay: "2024-12-01T00:00:00" });_13// Delay using a Date object_13await myTask.trigger(_13 { some: "data" },_13 { delay: new Date(Date.now() + 1000 * 60 * 60) }_13);
You can also pass a delay
option when batch triggering tasks:
_10await myTask.batchTrigger([_10 { payload: { some: "data" }, options: { delay: "1h" } },_10 { payload: { some: "data" }, options: { delay: "2h" } },_10 { payload: { some: "data" }, options: { delay: "15m" } },_10]);
You can also reschedule a task that has a delay set by calling runs.reschedule
:
_10import { runs } from "@trigger.dev/sdk/v3";_10_10// The delay option here takes the same format as the trigger delay option, and will be re-calculated from the current time_10await runs.reschedule("run_1234", { delay: "1h" });
TTL
You can set a TTL (time to live) when triggering a task, which will automatically expire the run if it hasn't started within the specified time. This is useful for ensuring that a run doesn't get stuck in the queue for too long, or for time-sensitive tasks.
NOTE
With this release, we're now setting a default TTL on development runs to 10m. This will prevent the issue where running the trigger.dev dev
CLI command after not using it for awhile would result in an avalanche of built-up runs.
_10import { myTask } from "./trigger/myTasks";_10_10// Expire the run if it hasn't started within 1 hour_10await myTask.trigger({ some: "data" }, { ttl: "1h" });_10_10// If you specify a number, it will be treated as seconds_10await myTask.trigger({ some: "data" }, { ttl: 3600 }); // 1 hour
Delayed runs with TTL
When you use both delay
and ttl
, the TTL will start counting down from the time the run is enqueued, not from the time the run is triggered.
So for example, when using the following code:
_10await myTask.trigger({ some: "data" }, { delay: "10m", ttl: "1h" });
The timeline would look like this:
- The run is created at 12:00:00
- The run is enqueued at 12:10:00
- The TTL starts counting down from 12:10:00
- If the run hasn't started by 13:10:00, it will be expired
For this reason, the ttl
option only accepts durations and not absolute timestamps.