Changelog #71
Declare cron on your tasks
You can now add cron patterns directly on your tasks – they'll sync in development and deployment.
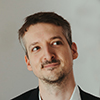
CEO, Trigger.dev
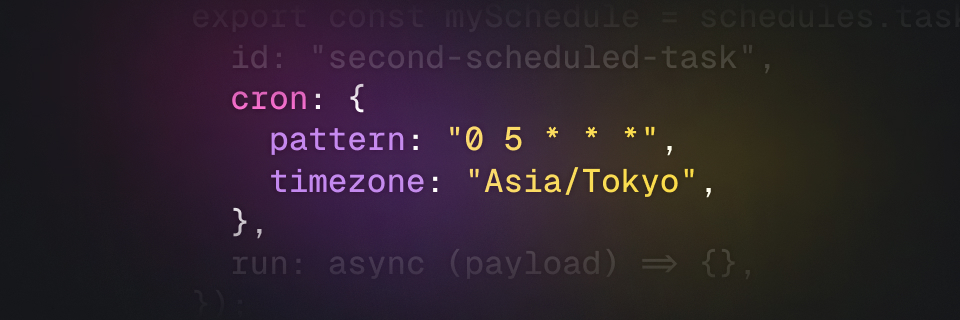
You've been able to add recurring schedules to your v3 tasks for a while now. You can attach schedules to a schedules.task
in the dashboard and dynamically using the SDK. This allows you to build some powerful things like recurring tasks for your users (using externalId
).
But what if you want a scheduled task with an attached CRON pattern in your code that syncs when you make changes? That's our new declarative CRON feature.
Declaring a CRON pattern
_11import { logger, schedules } from "@trigger.dev/sdk/v3";_11_11export const checkMetrics = schedules.task({_11 id: "check-metrics",_11 //6am every day_11 cron: "0 6 * * *",_11 run: async (payload) => {_11 const formattedTime = payload.timestamp.toLocaleString("en-US");_11 logger.log(formattedTime);_11 },_11});
That cron
property is an optional property you can add to a schedules.task
. If you leave it off then schedules work as before (you add them explicitly in the dashboard or using the SDK).
If you add it then a schedule will be synced whenever you run the dev or deploy commands with the CLI (including in GitHub Actions). We sync when the cron property is added, updated, or removed.
With a timezone
UTC can be painful to work with so we also support adding a timezone:
_18import { logger, schedules } from "@trigger.dev/sdk/v3";_18_18export const checkMetrics = schedules.task({_18 id: "check-metrics",_18 //6am every day in New York_18 cron: {_18 pattern: "0 6 * * *",_18 timezone: "America/New_York",_18 },_18 run: async (payload) => {_18 const formattedTime = payload.timestamp.toLocaleString("en-US", {_18 //use the timezone to format the time_18 timeZone: payload.timezone,_18 });_18_18 logger.log(formattedTime);_18 },_18});
Just like when specifying the timezone using our imperative functions, this respects Daylight Saving Time changes. You can see a full list of timezones we support.
Declarative vs Imperative schedules
This new type of CRON schedule is "declarative" and our existings one are "imperative".
Declarative schedules can only be created, updated, or removed by editing your schedules.task
cron
property in your code and then syncing by running dev
or deploy
. You cannot modify them in the dashboard or using the imperative SDK functions.
Each environment gets a separate declarative schedule – if you run the dev and deploy commands you'll end up with two schedules in the dashboard (one for dev and one for prod). If you run deploy with the staging flag then you'll get another. This means you won't accidentally alter a production schedule when you're working in dev.
Generally we recommend using declarative schedules for your internal tasks and imperative schedules for your user-facing tasks. This is because declarative schedules are easier to manage and track in your codebase.
Read the full docs for all the options and functions available on schedules.