Next.js setup guide
This guide will show you how to setup Trigger.dev in your existing Next.js project, test an example task, and view the run.
Prerequisites
- Setup a project in
- Ensure TypeScript is installed
- Create a Trigger.dev account
- Create a new Trigger.dev project
Initial setup
Run the CLI `init` command
The easiest way to get started is to use the CLI. It will add Trigger.dev to your existing project, create a /trigger
folder and give you an example task.
Run this command in the root of your project to get started:
It will do a few things:
- Log you into the CLI if you’re not already logged in.
- Create a
trigger.config.ts
file in the root of your project. - Ask where you’d like to create the
/trigger
directory. - Create the
/trigger
directory with an example task,/trigger/example.[ts/js]
.
Install the “Hello World” example task when prompted. We’ll use this task to test the setup.
Run the CLI `dev` command
The CLI dev
command runs a server for your tasks. It watches for changes in your /trigger
directory and communicates with the Trigger.dev platform to register your tasks, perform runs, and send data back and forth.
It can also update your @trigger.dev/*
packages to prevent version mismatches and failed deploys. You will always be prompted first.
Perform a test run using the dashboard
The CLI dev
command spits out various useful URLs. Right now we want to visit the Test page
You should see our Example task in the list
, select it. Most tasks have a “payload” which you enter in the JSON editor , but our example task doesn’t need any input.Press the “Run test” button
.View your run
Congratulations, you should see the run page which will live reload showing you the current state of the run.
If you go back to your terminal you’ll see that the dev command also shows the task status and links to the run log.
Set your secret key locally
Set your TRIGGER_SECRET_KEY
environment variable in your .env.local
file if using the Next.js App router or .env
file if using Pages router. This key is used to authenticate with Trigger.dev, so you can trigger runs from your Next.js app. Visit the API Keys page in the dashboard and select the DEV secret key.
For more information on authenticating with Trigger.dev, see the API keys page.
Triggering your task in Next.js
Here are the steps to trigger your task in the Next.js App and Pages router and Server Actions. Alternatively, check out this repo for a full working example of a Next.js app with a Trigger.dev task triggered using a Server Action.
Create a Route Handler
Add a Route Handler by creating a route.ts
file (or route.js
file) in the app/api
directory like this: app/api/hello-world/route.ts
.
Add your task
Add this code to your route.ts
file which imports your task along with NextResponse
to handle the API route response:
Trigger your task
Run your Next.js app:
Run the dev server from Step 2. of the Initial Setup section above if it’s not already running:
Now visit the URL in your browser to trigger the task. Ensure the port number is the same as the one you’re running your Next.js app on. For example, if you’re running your Next.js app on port 3000, visit:
You should see the CLI log the task run with a link to view the logs in the dashboard.
Visit the Trigger.dev dashboard to see your run.
Automatically sync environment variables from your Vercel project (optional)
If you want to automatically sync environment variables from your Vercel project to Trigger.dev, you can add our syncVercelEnvVars
build extension to your trigger.config.ts
file.
You need to set the VERCEL_ACCESS_TOKEN
and VERCEL_PROJECT_ID
environment variables, or pass
in the token and project ID as arguments to the syncVercelEnvVars
build extension. If you’re
working with a team project, you’ll also need to set VERCEL_TEAM_ID
, which can be found in your
team settings. You can find / generate the VERCEL_ACCESS_TOKEN
in your Vercel
dashboard. Make sure the scope of the token covers
the project with the environment variables you want to sync.
For more information, see our Vercel sync environment variables guide.
Manually add your environment variables (optional)
If you have any environment variables in your tasks, be sure to add them in the dashboard so deployed code runs successfully. In Node.js, these environment variables are accessed in your code using process.env.MY_ENV_VAR
.
In the sidebar select the “Environment Variables” page, then press the “New environment variable” button.
You can add values for your local dev environment, staging and prod.
You can also add environment variables in code by following the steps on the Environment Variables page.
Deploying your task to Trigger.dev
For this guide, we’ll manually deploy your task by running the CLI deploy command below. Other ways to deploy are listed in the next section.
Other ways to deploy
Use GitHub Actions to automatically deploy your tasks whenever new code is pushed and when the trigger
directory has changes in it. Follow this guide to set up GitHub Actions.
Troubleshooting & extra resources
Revalidation from your Trigger.dev tasks
Revalidation allows you to purge the cache for an ISR route. To revalidate an ISR route from a Trigger.dev task, you have to set up a handler for the revalidate
event. This is an API route that you can add to your Next.js app.
This handler will run the revalidatePath
function from Next.js, which purges the cache for the given path.
The handlers are slightly different for the App and Pages router:
Revalidation handler: App Router
If you are using the App router, create a new revalidation route at app/api/revalidate/path/route.ts
:
Revalidation handler: Pages Router
If you are using the Pages router, create a new revalidation route at pages/api/revalidate/path.ts
:
Revalidation task
This task takes a path
as a payload and will revalidate the path you specify, using the handler you set up previously.
To run this task locally you will need to set the REVALIDATION_SECRET
environment variable in your .env.local
file (or .env
file if using Pages router).
To run this task in production, you will need to set the REVALIDATION_SECRET
environment variable in Vercel, in your project settings, and also in your environment variables in the Trigger.dev dashboard.
Testing the revalidation task
You can test your revalidation task in the Trigger.dev dashboard on the testing page, using the following payload.
Next.js build failing due to missing API key in GitHub CI
This issue occurs during the Next.js app build process on GitHub CI where the Trigger.dev SDK is expecting the TRIGGER_SECRET_KEY environment variable to be set at build time. Next.js attempts to compile routes and creates static pages, which can cause issues with SDKs that require runtime environment variables. The solution is to mark the relevant pages as dynamic to prevent Next.js from trying to make them static. You can do this by adding the following line to the route file:
Correctly passing event handlers to React components
An issue can sometimes arise when you try to pass a function directly to the onClick
prop. This is because the function may require specific arguments or context that are not available when the event occurs. By wrapping the function call in an arrow function, you ensure that the handler is called with the correct context and any necessary arguments. For example:
This works:
Whereas this does not work:
Learn more about Next.js and Trigger.dev
Walk-through guides from development to deployment
Next.js - setup guide
Learn how to setup Trigger.dev with Next.js, using either the pages or app router.
Next.js - triggering tasks using webhooks
Learn how to create a webhook handler for incoming webhooks in a Next.js app, and trigger a task from it.
Task examples
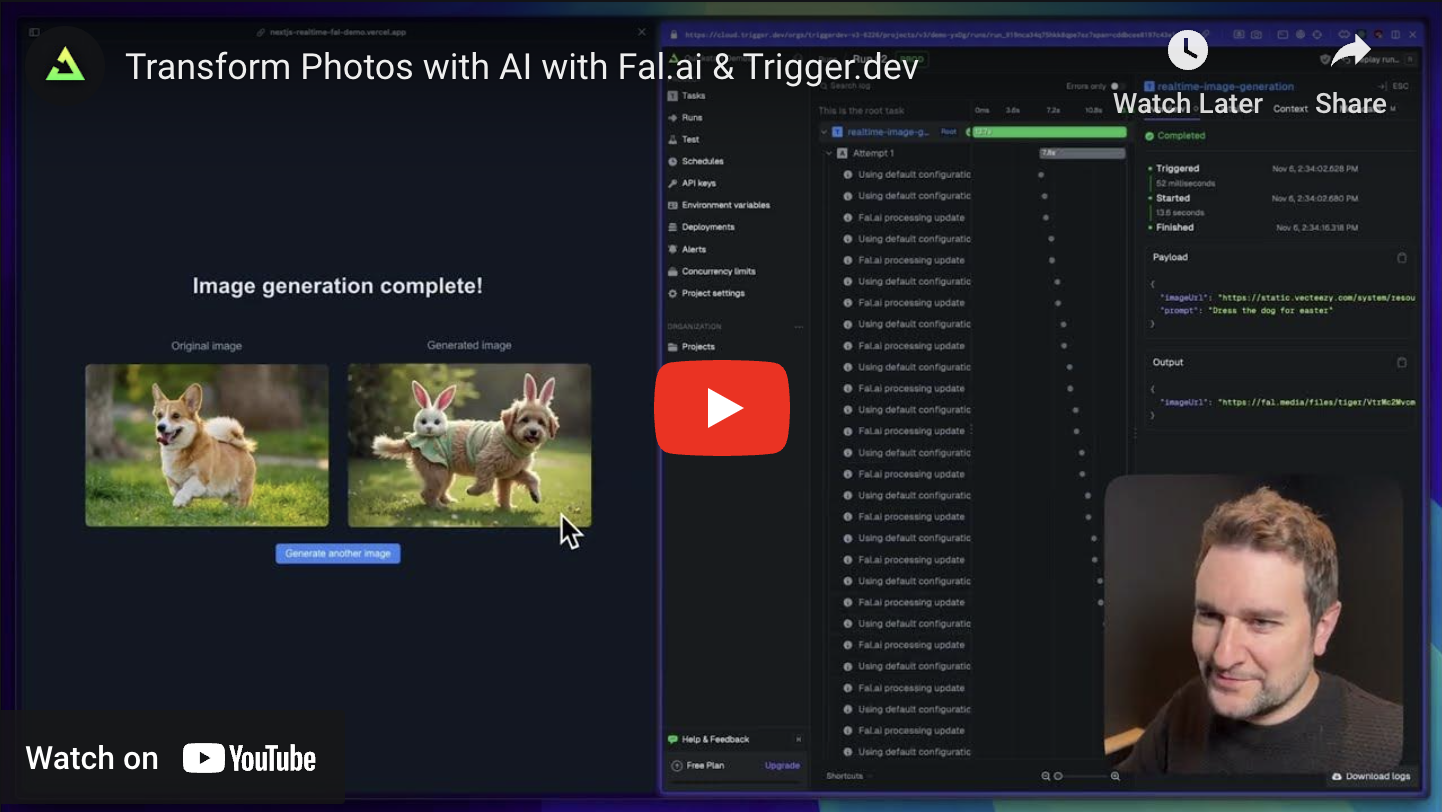
Fal.ai with Realtime in Next.js
Generate an image from a prompt using Fal.ai and Trigger.dev Realtime.
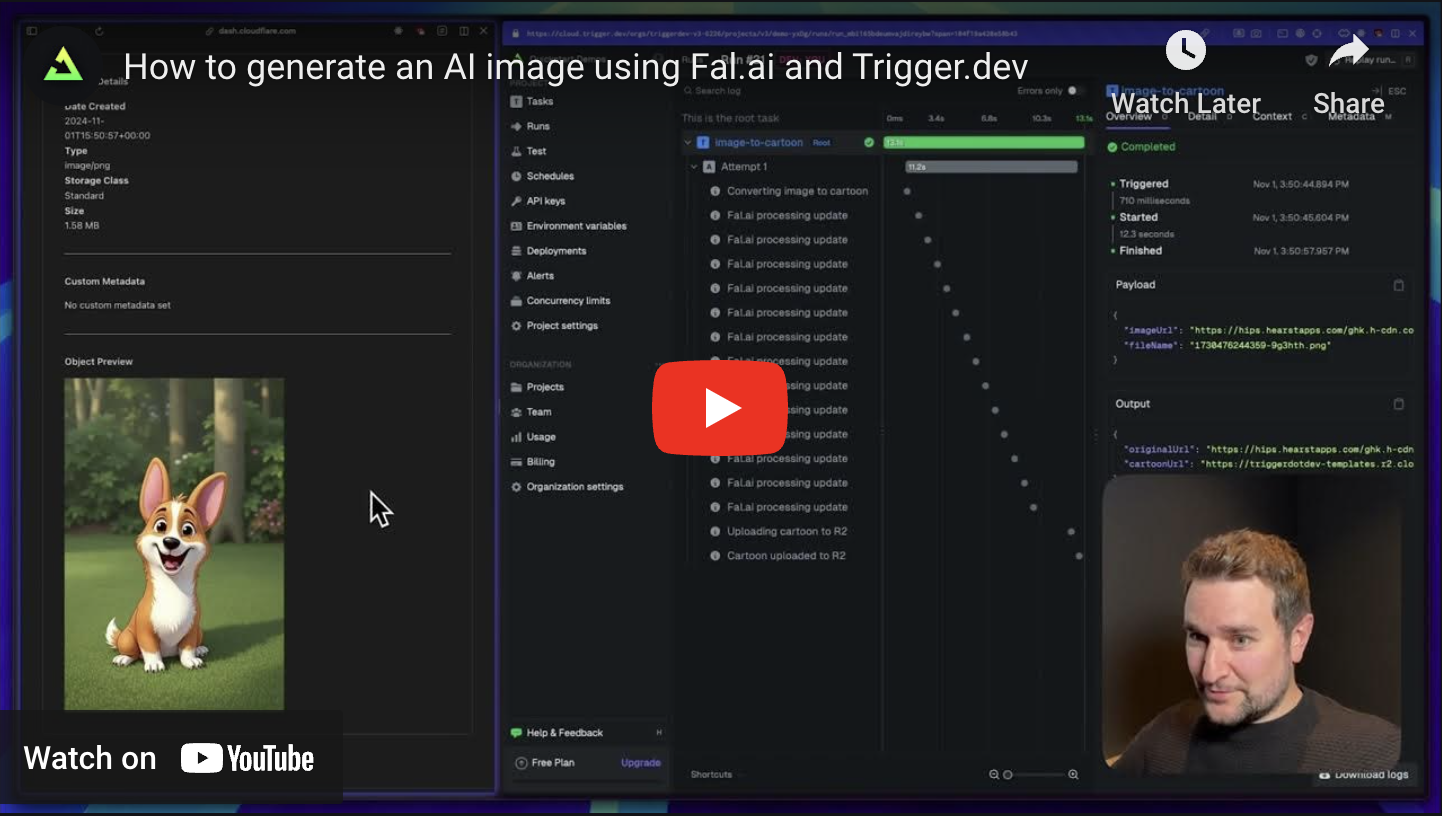
Generate a cartoon using Fal.ai in Next.js
Convert an image to a cartoon using Fal.ai.
Vercel sync environment variables
Learn how to automatically sync environment variables from your Vercel projects to Trigger.dev.
Vercel AI SDK
Learn how to use the Vercel AI SDK, which is a simple way to use AI models from different providers, including OpenAI, Anthropic, Amazon Bedrock, Groq, Perplexity etc.
Useful next steps
Was this page helpful?