Changelog #64
runs.get() & runs.list()
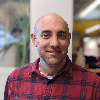
CTO, Trigger.dev
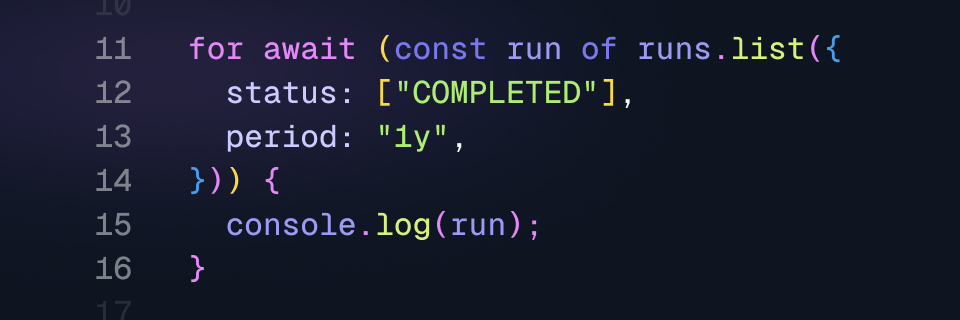
We've added first-class support for getting runs using the SDK in version 3.0.0-beta.36
. The list
function is especially nice as we're using some pretty neat tricks to make it really easy to paginate through runs.
If you haven't used any of the management functions before, the overview which includes instructions on authentication is a good starting point.
Run this command in your repo to easily upgrade:
_10npx trigger.dev@beta update
runs.retrieve()
_16import { runs, APIError } from "@trigger.dev/sdk/v3";_16_16//run IDs start with "run_"_16async function getRun(id: string) {_16 try {_16 const run = await runs.retrieve(id);_16 } catch (error) {_16 if (error instanceof ApiError) {_16 console.error(_16 `API error: ${error.status}, ${error.headers}, ${error.body}`_16 );_16 } else {_16 console.error(`Unknown error: ${error.message}`);_16 }_16 }_16}
You get lots of useful information about the run including:
status
: (e.g.QUEUED
,EXECUTING
,COMPLETED
,FAILED
, etc)payload
: The payload that was sent to the runoutput
: The output of the run, if it has completedstartedAt
: When the run was startedfinishedAt
: When the run finished, if it has completedattempts
: Information about each attempt, including the status and any error.
runs.list()
Auto-pagination
Dealing with list pagination is usually a pain. You get a bunch of items back, then you have to make another request to get the next page, and so on. We've made this super easy with the list
function. You can just use a for await
loop to iterate through every single matching run.
_11import { runs } from "@trigger.dev/sdk/v3";_11_11async function fetchAllRuns() {_11 const allRuns = [];_11_11 for await (const run of runs.list({ limit: 10 })) {_11 allRuns.push(run);_11 }_11_11 return allRuns;_11}
Be warned: this will go through every single run, so you should break out of the loop when you have what you need. Or at least use a filter to only get the runs you're interested in.
Using getNextPage()
Alternatively, you can get a page then use getNextPage()
to get the next page.
_14import { runs } from "@trigger.dev/sdk/v3";_14_14async function fetchRuns() {_14 let page = await runs.list({ limit: 10 });_14_14 for (const run of page.data) {_14 console.log(run);_14 }_14_14 while (page.hasNextPage()) {_14 page = await page.getNextPage();_14 // ... do something with the next page_14 }_14}
Filtering
You can use advanced filtering when listing runs. For example, to get all completed runs in the last year:
_10for await (const run of runs.list({_10 status: ["COMPLETED"],_10 period: "1y",_10})) {_10 console.log(run);_10}
Or just encode-video
tasks in the past day, that aren't tests and failed:
_10for await (const run of runs.list({_10 taskIdentifier: "encode-video",_10 status: ["FAILED", "CRASHED", "INTERRUPTED", "SYSTEM_FAILURE"],_10 period: "1d",_10 isTest: false,_10})) {_10 console.log(run);_10}