Changelog #78
Run metadata
Attach a small amount of data to a run and update it as the run progresses.
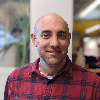
CTO, Trigger.dev
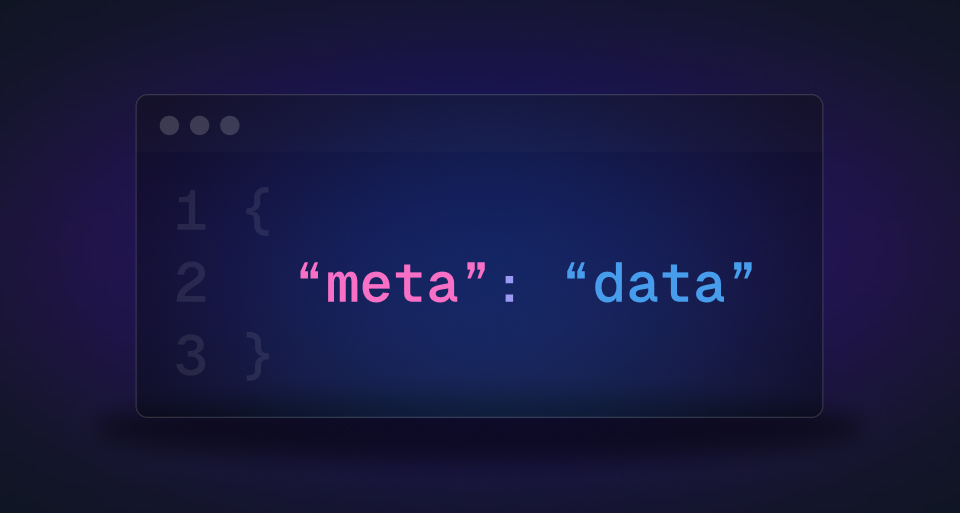
You can now attach up to 4KB (4,096 bytes) of metadata to a run, which you can then access from inside the run function, via the API, and in the dashboard. You can use metadata to store additional, structured information on a run. For example, you could use metadata to update the progress of a run.
Usage
Add metadata to a run by passing it as an object to the trigger
function:
_10const handle = await myTask.trigger(_10 { message: "hello world" },_10 { metadata: { user: { name: "Eric", id: "user_1234" } } }_10);
Then inside your run function, you can access the metadata like this:
_10import { task, metadata } from "@trigger.dev/sdk/v3";_10_10export const myTask = task({_10 id: "my-task",_10 run: async (payload: { message: string }) => {_10 const user = metadata.get("user");_10 console.log(user.name); // "Eric"_10 console.log(user.id); // "user_1234"_10 },_10});
You can also update the metadata during the run:
_34import { task, metadata } from "@trigger.dev/sdk/v3";_34_34export const myTask = task({_34 id: "my-task",_34 run: async (payload: { message: string }) => {_34 // Do some work_34 await metadata.set("status", {_34 state: "running",_34 progress: 0.1,_34 message: "Starting",_34 });_34_34 // Do some more work_34 await metadata.set("status", {_34 state: "running",_34 progress: 0.5,_34 message: "Halfway there",_34 });_34_34 // Do even more work_34 await metadata.set("status", {_34 state: "running",_34 progress: 0.9,_34 message: "Almost done",_34 });_34_34 // Finish up_34 await metadata.set("status", {_34 state: "complete",_34 progress: 1,_34 message: "Done",_34 });_34 },_34});
Access the metadata outside of a task via the API:
_10// Somewhere in your backend code_10import { runs } from "@trigger.dev/sdk/v3";_10_10async function main() {_10 const run = await runs.retrieve("run_1234");_10 console.log(run.metadata.user.name); // "Eric"_10 console.log(run.metadata.user.id); // "user_1234"_10}
Learn all about how to use metadata in the metadata documentation.
Updating
Run metadata is available as of version 3.0.8
of the SDK. To update, run:
_10npx trigger.dev@latest update
If you are self-hosting Trigger.dev, you will need to update to version 3.0.8 or later. See our self-hosting updating docs for more information.