Changelog #53
Scheduled tasks for v3
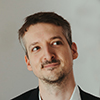
CEO, Trigger.dev
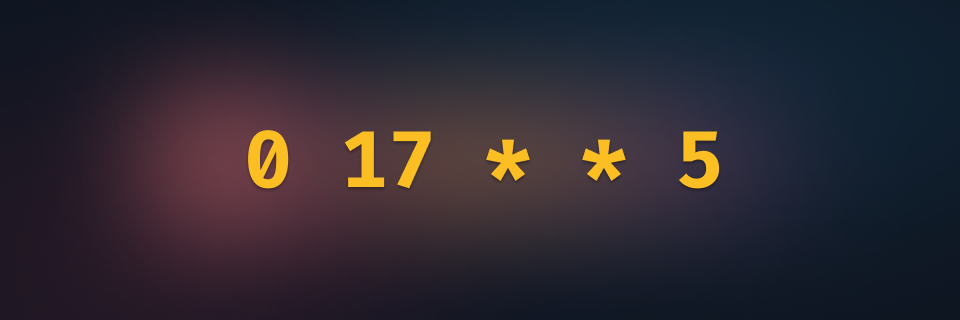
Now you can create scheduled tasks in Trigger.dev v3. If you haven't already you should sign up for the v3 waitlist.
At the end I'll reveal what schedule the CRON pattern in the image above, 0 17 * * 5
, represents. You CRON nerds need closure.
In the video my scheduled task posts a GIF to Slack every minute.
Features
- Define your task in code using
schedules.task()
- Attach many schedules (across environments) to each task in the dashboard.
- Attach schedules using the SDK (you can do dynamic things like a schedule for each of your users using
externalId
) - List, view, edit, disable, re-enable, create and delete using the dashboard, SDK and REST API.
- Use AI to help you create CRON patterns in the dashboard.
Full docs here: https://trigger.dev/docs/v3/tasks-scheduled
An example scheduled task
This is the scheduled task code from the video above.
As shown in the video, you can attach a schedule to this task in the dashboard. This is great for most use cases.
Dynamic schedules (or multi-tenant)
Using the SDK you can do more advanced scheduling. For example, you could let your users define a schedule that they want to post GIFs to their own Slack workspace channel.
First take what we had before and modify the task slightly:
Then in your backend code you need to register a schedule for this task. This is a Next.js server action but the only thing that matters is that it's on your server:
_33"use server";_33_33import { scheduledSlackGifs } from "@/trigger/scheduled-user-gifs";_33import { schedules } from "@trigger.dev/sdk/v3";_33import { db } from "@/db";_33_33export async function registerSchedule(_33 userId: string,_33 cron: string,_33 searchQuery: string_33) {_33 try {_33 //create a record for the GIF schedule for this user_33 const row = await db.createGifSchedule(userId, searchQuery);_33_33 //create a new schedule for this GIF schedule_33 const createdSchedule = await schedules.create({_33 task: scheduledSlackGifs.id,_33 cron,_33 //the row id, so we can retrieve the row inside the run_33 externalId: row.id,_33 //don't allow multiple shedules for the same GIF schedule_33 deduplicationKey: row.id,_33 });_33_33 return { scheduleId: createdSchedule.id };_33 } catch (error) {_33 console.error(error);_33 return {_33 error: "something went wrong",_33 };_33 }_33}
The user has defined their own reminder frequency and what kind of GIFs they want.
Read the full docs for everything that scheduled tasks allow.
So the CRON pattern 0 17 * * 5
is every Friday at 5pm (UTC). I hope that helps you sleep at night.