Changelog #103
·
Run cleanup using onCancel hook
Define an onCancel hook that is called when a run is canceled
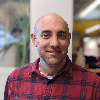
CTO, Trigger.dev
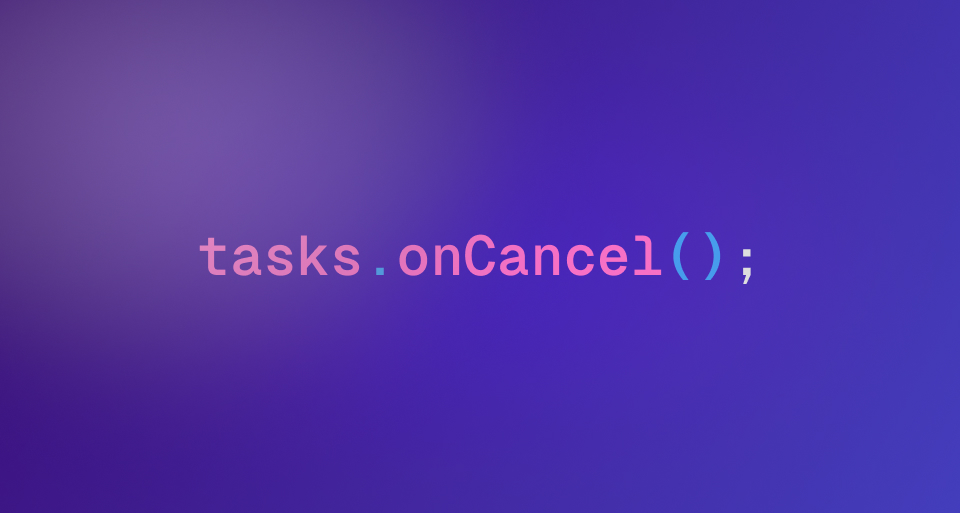
You can now define an onCancel
hook that is called when a run is canceled. This is useful if you want to clean up any resources that were allocated for the run.
tasks.onCancel(({ ctx, signal }) => { console.log("Run canceled", signal);});
The onCancel
hook can be used to interrupt calls to external services. For example, when using the streamText
function from the AI SDK:
import { logger, tasks, schemaTask } from "@trigger.dev/sdk";import { streamText } from "ai";import { z } from "zod";export const interruptibleChat = schemaTask({ id: "interruptible-chat", description: "Chat with the AI", schema: z.object({ prompt: z.string().describe("The prompt to chat with the AI"), }), run: async ({ prompt }, { signal }) => { const chunks: TextStreamPart<{}>[] = []; tasks.onCancel(async () => { // We have access to the chunks here, and can save them to the database await saveChunksToDatabase(chunks); }); try { const result = streamText({ model: getModel(), prompt, experimental_telemetry: { isEnabled: true, }, tools: {}, abortSignal: signal, // Pass the signal to the streamText function onChunk: ({ chunk }) => { chunks.push(chunk); }, }); const textParts = []; for await (const part of result.textStream) { textParts.push(part); } return textParts.join(""); } catch (error) { if (error instanceof Error && error.name === "AbortError") { // Handle abort error } else { throw error; } } },});
You can also use the onCancel
hook to wait for the run function to finish and access its output:
import { logger, task } from "@trigger.dev/sdk";import { setTimeout } from "node:timers/promises";export const cancelExampleTask = task({ id: "cancel-example", run: async (payload: { message: string }, { signal }) => { try { await setTimeout(10_000, undefined, { signal }); } catch (error) { // Ignore the abort error } return { message: "Hello, world!", }; }, onCancel: async ({ runPromise }) => { // You can await the runPromise to get the output of the task const output = await runPromise; },});
Note: You have up to 30 seconds to complete the runPromise
in the onCancel
hook. After that point the process will be killed.
This feature is only available in v4.0.0-beta.12 and later.