Changelog #44
·
Key-Value Store
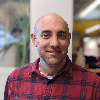
CTO, Trigger.dev
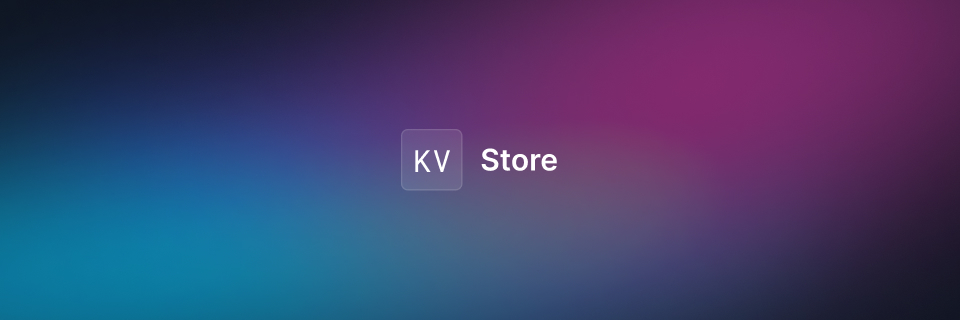
The Key-Value Store enables you to store and retrieve small chunks of serializable data in and outside of your Jobs.
Use the io.store
object to access namespaced stores inside of your Run functions. Or use client.store
to access the environment store anywhere in your app!
Sharing data across Runs with io.store.job
:
_13client.defineJob({_13 ..._13 run: async (payload, io, ctx) => {_13 // this will only be undefined on the first run_13 const counter = await io.store.job.get<number>("job-get", "counter")_13_13 const currentCount = counter ?? 0_13 const incrementedCounter = currentCount++_13_13 // Job-scoped set_13 await io.store.job.set("job-set", "counter", incrementedCounter);_13 }_13})
Sharing data across Jobs with io.store.env
:
_20client.defineJob({_20 id: "job-1",_20 ..._20 run: async (payload, io, ctx) => {_20 // store data in one job_20 await io.store.env.set("cacheKey", "cross-run-shared-key", { foo: "bar" });_20 }_20})_20_20client.defineJob({_20 id: "job-2",_20 ..._20 run: async (payload, io, ctx) => {_20 // access from a different job_20 const value = await io.store.env.get<{ foo: string }>("cacheKey", "cross-run-shared-key");_20 }_20})_20_20// or anywhere else in your app_20await client.store.env.get<{ foo: string }>("cross-run-shared-key");
Full docs for io.store and client.store.