Changelog #33
Invoke Trigger
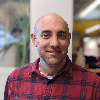
CTO, Trigger.dev
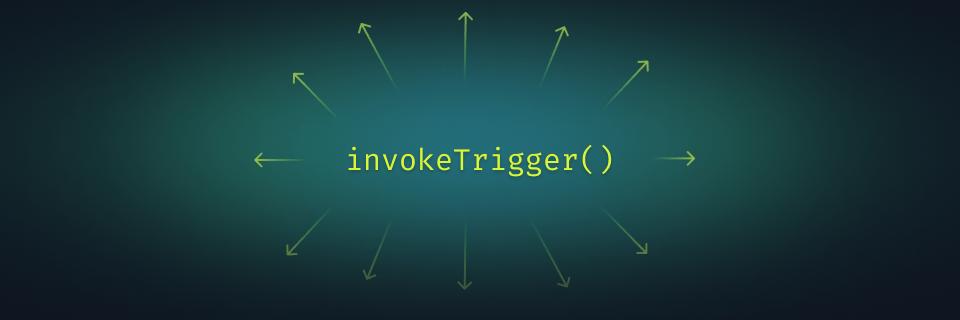
Up until now, Trigger.dev only supported the following 3 types of triggers: Events, Webhooks, and Scheduled (e.g. cron/interval).
But sometimes it makes sense to be able to invoke a Job manually, without having to specify an event, especially for cases where you want to get notified when the invoked Job Run is complete.
To specify that a job is manually invokable, you can use the invokeTrigger()
function when defining a job:
_16import { invokeTrigger } from "@trigger.dev/sdk";_16import { client } from "@/trigger";_16_16export const exampleJob = client.defineJob({_16 id: "example-job",_16 name: "Example job",_16 version: "1.0.1",_16 trigger: invokeTrigger({_16 schema: z.object({_16 foo: z.string(),_16 }),_16 }),_16 run: async (payload, io, ctx) => {_16 // do something with the payload_16 },_16});
And then you can invoke the job using the Job.invoke()
method:
_10import { exampleJob } from "./exampleJob";_10_10const jobRun = await exampleJob.invoke(_10 { foo: "bar" },_10 { callbackUrl: `${process.env.VERCEL_URL}/api/callback` }_10);
Which is great but things become really cool when you invoke a job from another job and wait for the invoked job to complete:
_15import { exampleJob } from "./exampleJob";_15_15client.defineJob({_15 id: "example-job2",_15 name: "Example job 2",_15 version: "1.0.1",_15 trigger: intervalTrigger({_15 seconds: 60,_15 }),_15 run: async (payload, io, ctx) => {_15 const runResult = await exampleJob.invokeAndWaitForCompletion("⚡", {_15 foo: "123",_15 });_15 },_15});
You can also batch up to 25 invocations at once, and we will run them in parallel and wait for all of them to complete before continuing execution of the current job.
_28import { exampleJob } from "./exampleJob";_28_28client.defineJob({_28 id: "example-job2",_28 name: "Example job 2",_28 version: "1.0.1",_28 trigger: intervalTrigger({_28 seconds: 60,_28 }),_28 run: async (payload, io, ctx) => {_28 const runs = await exampleJob.batchInvokeAndWaitForCompletion("⚡", [_28 {_28 payload: {_28 userId: "123",_28 tier: "free",_28 },_28 },_28 {_28 payload: {_28 userId: "abc",_28 tier: "paid",_28 },_28 },_28 ]);_28_28 // runs is an array of RunNotification objects_28 },_28});
See our Invoke Trigger docs for more info. Upgrade to the latest version of the SDK to start using this feature.
How to update
The Trigger.dev Cloud is now running v2.2.4
. If you are self-hosting you can upgrade by pinning to the v2.2.4
tag.
The trigger.dev/*
packages are now at v2.2.5
. You can update using the following command:
_10npx @trigger.dev/cli@latest update