Blog
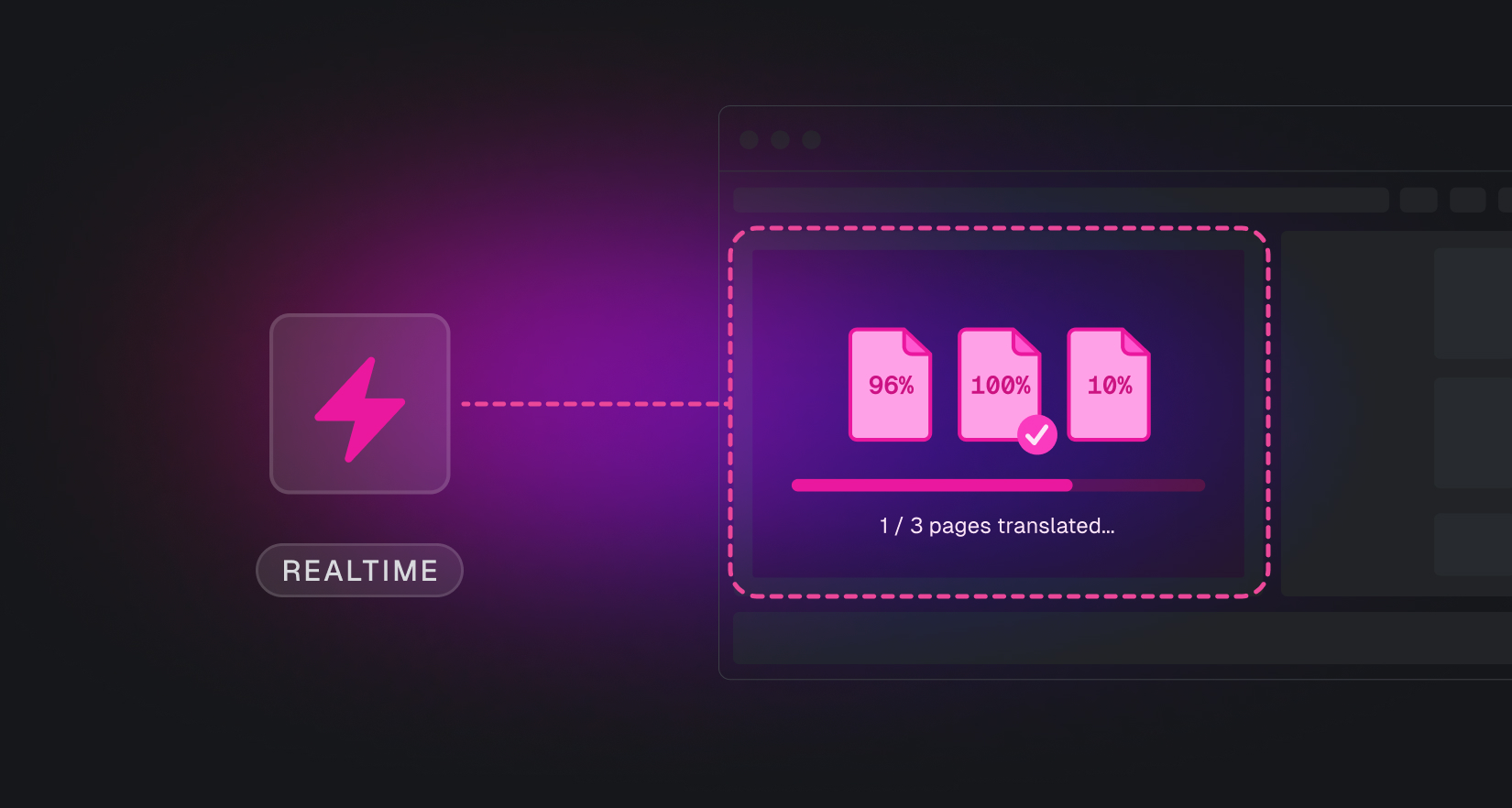
Article
How we built a real-time service that handles 20,000 updates per second
We process 20,000 run updates per second and 500GB of data daily using Postgres replication slots and ElectricSQL. Here's why we chose this over WebSockets and how we solved the hard problems of authentication, rate limiting, and caching.
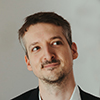
Matt Aitken
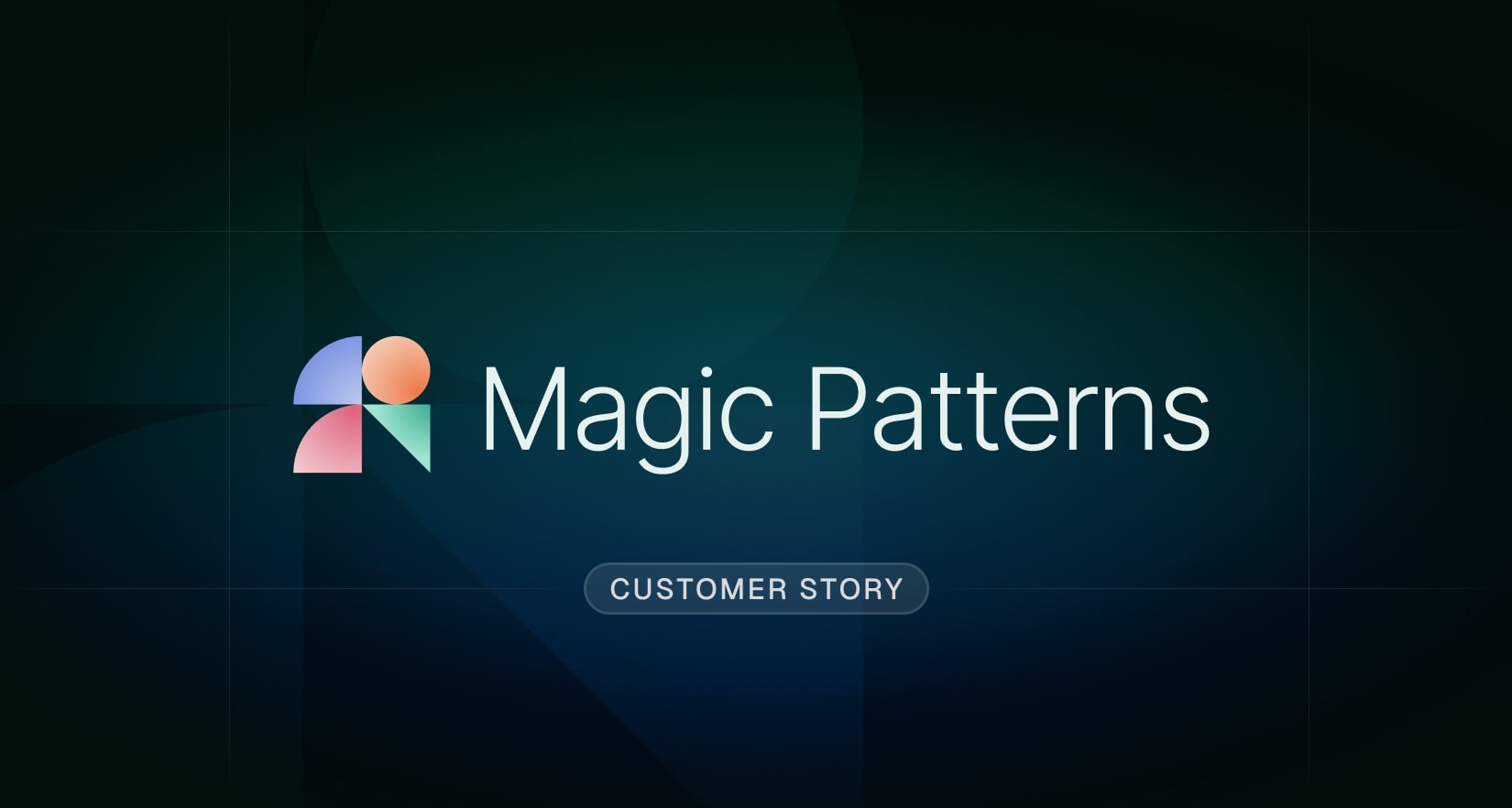
Customer story
How Magic Patterns migrated 200k monthly jobs to Trigger.dev in one day
Alex Danilowicz, Co-founder of Magic Patterns, shares why they migrated their critical background jobs to Trigger.dev from their unreliable self-hosted solution. As a small team serving enterprise clients like PwC and Lendi Group, they needed reliable infrastructure that wouldn't consume their limited engineering resources.
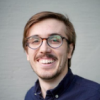
Alex Danilowicz
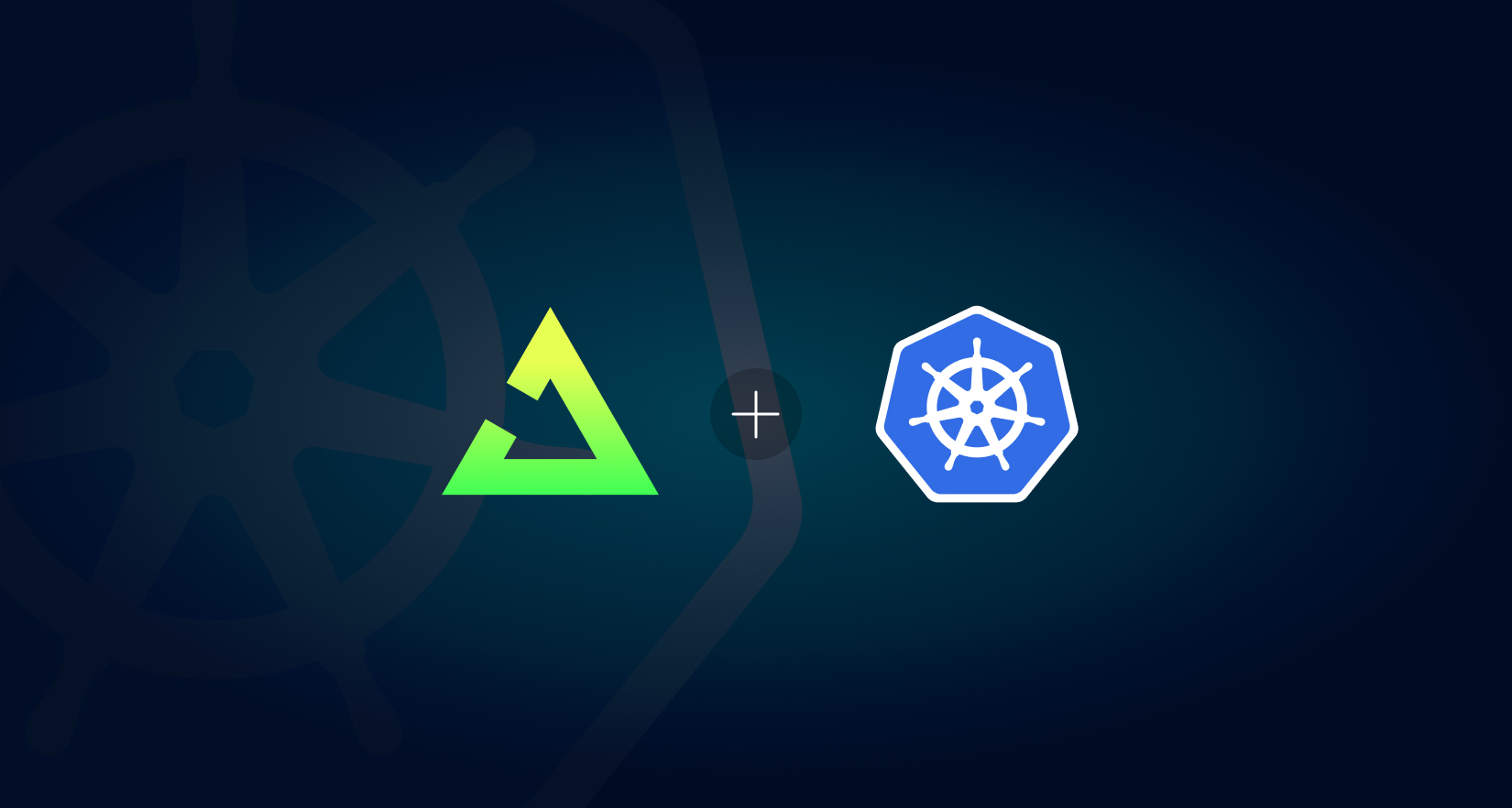
Article
Self-hosting Trigger.dev v4 using Kubernetes
You can now self-host Trigger.dev v4 using Kubernetes. This adds to the existing Docker option.
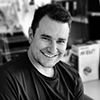
James Ritchie
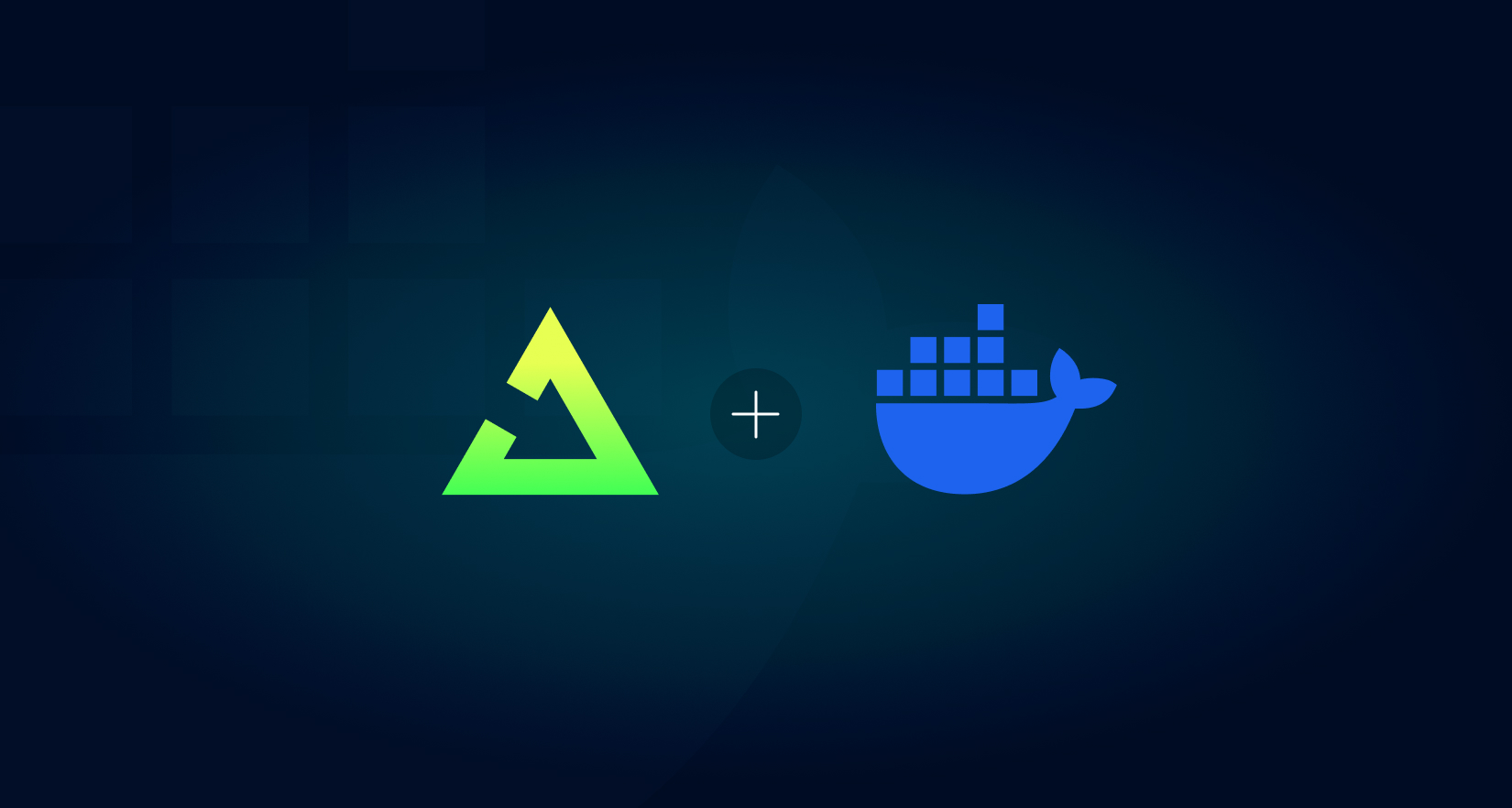
Article
Self-hosting Trigger.dev v4 using Docker
You can now self-host Trigger.dev v4 using Docker. This is a big step towards making Trigger.dev more accessible to everyone.
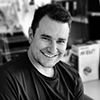
James Ritchie
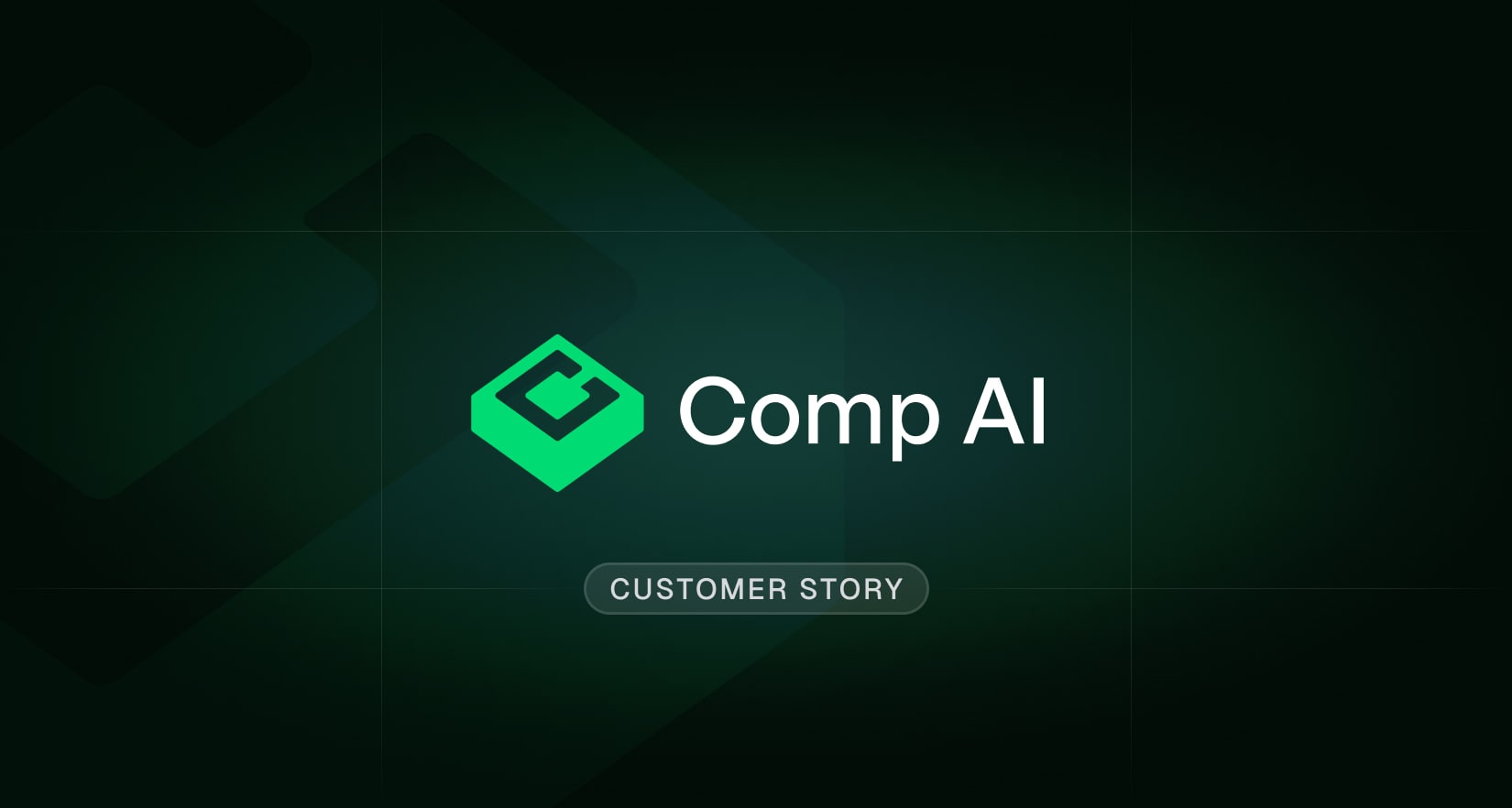
Customer story
Automating security compliance using AI agents
Lewis Carhart, Founder at Comp AI, shares how they use Trigger.dev to automate evidence collection at scale, powering their open source, AI-driven compliance platform.
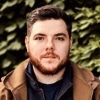
Lewis Carhart
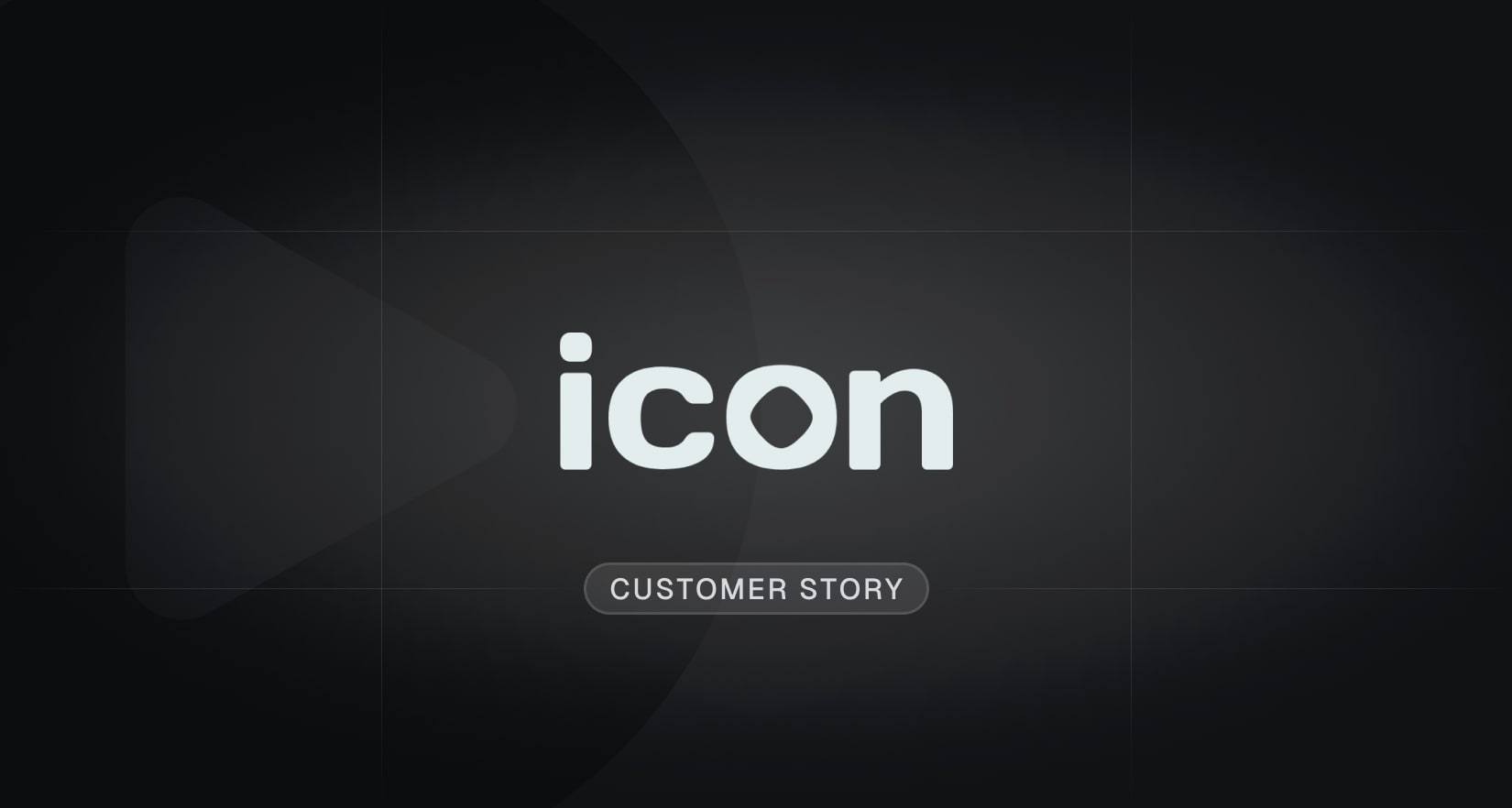
Customer story
Icon: revolutionizing video ad creation with Trigger.dev
Caleb Tan, Founding Engineer at Icon, shares how they use Trigger.dev to process thousands of videos concurrently, powering their AI-driven ad creation platform that allows global brands to generate full video ads in minutes.
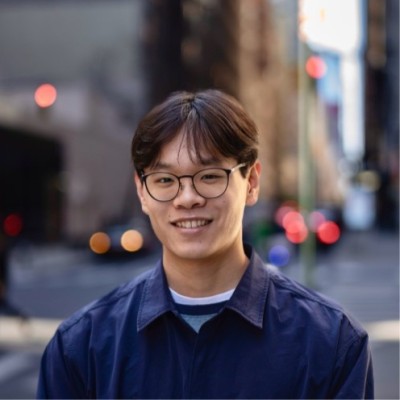
Caleb Tan
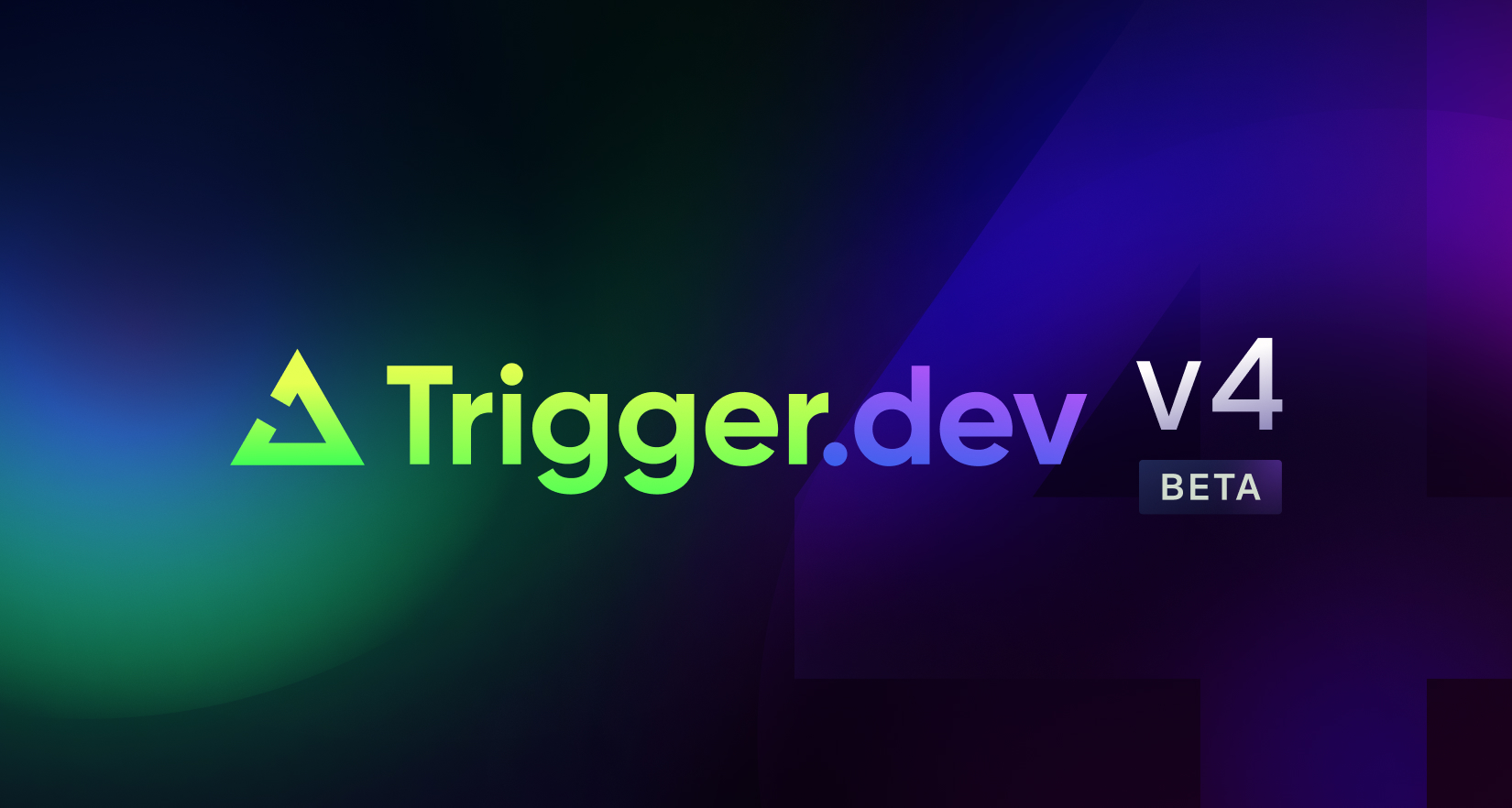
Announcement
Trigger.dev v4 beta
Today we're excited to launch our v4 beta, that includes Run Engine 2. There are significant performance improvements like warm starts, a totally revamped dashboard, human in the loop tokens, and more.
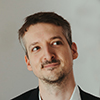
Matt Aitken
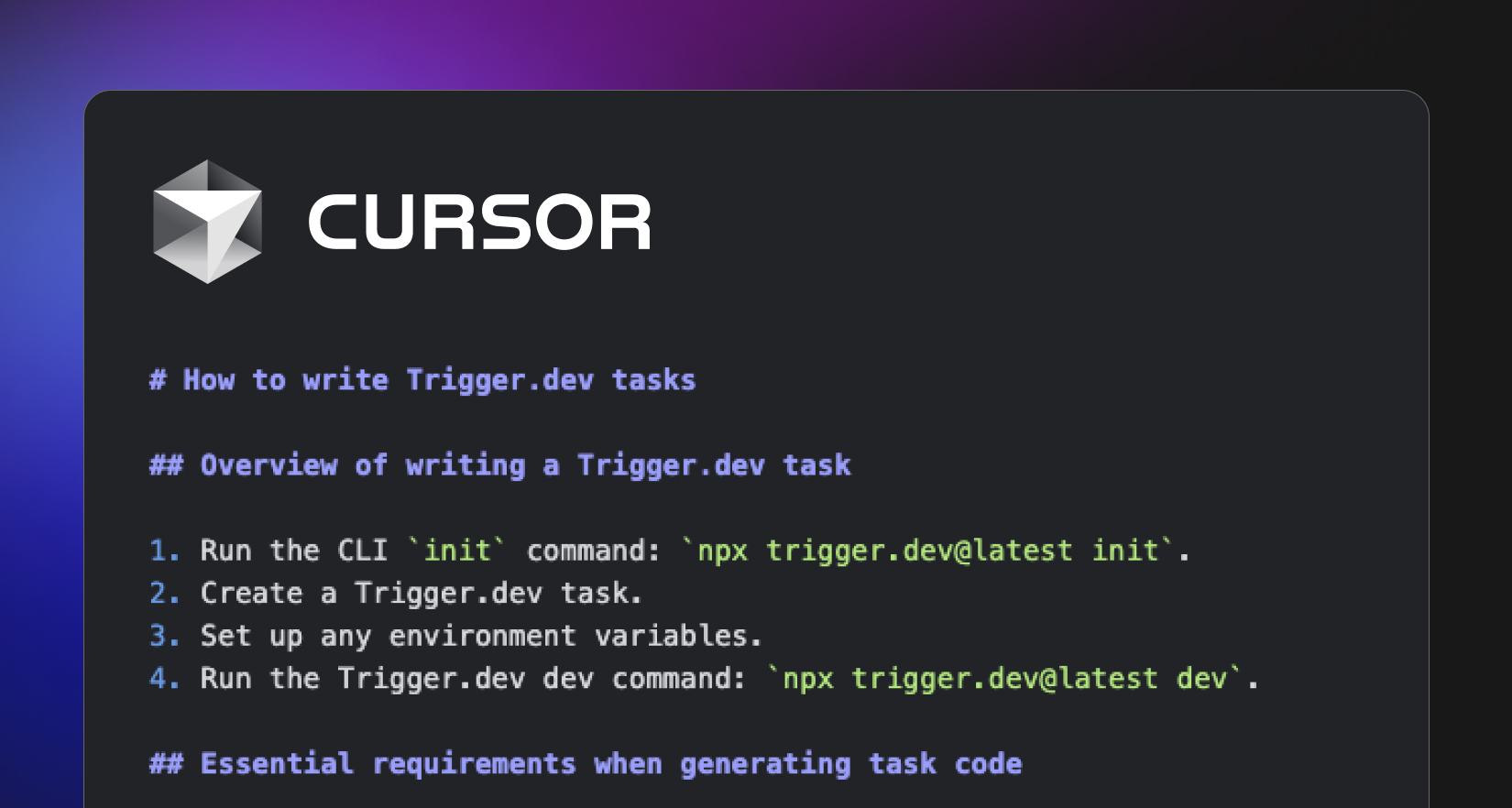
Article
How to write great Cursor Rules
What we learned writing a Cursor Rules file for Trigger.dev tasks, and 10 tips for writing your own
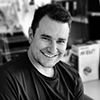
James Ritchie
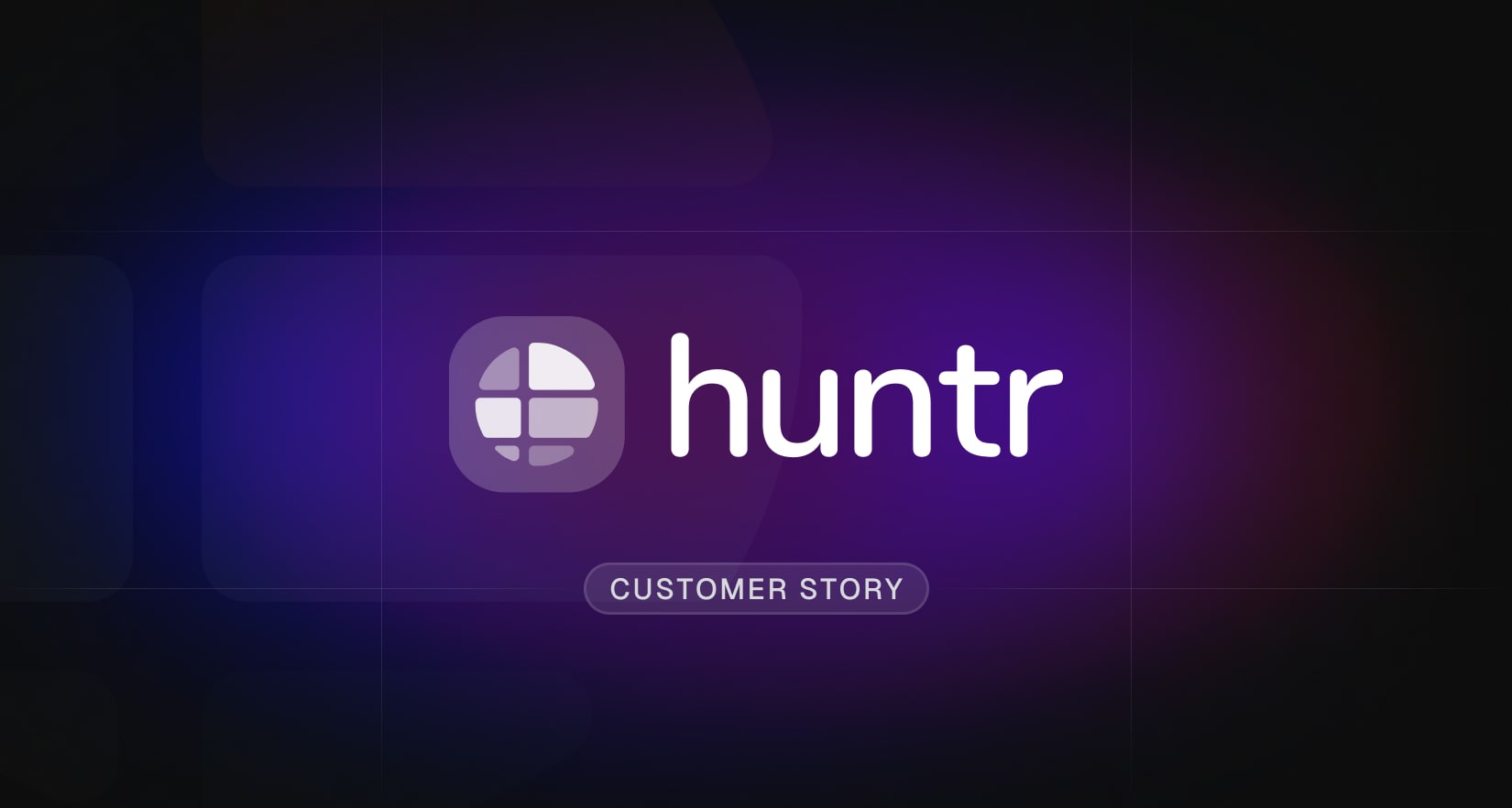
Customer story
How Trigger.dev powers Huntr’s internal AI agents
Sam Wright, Head of Operations and Partnerships at Huntr, explains how they use Trigger.dev to build AI agents for content generation and internal tooling.
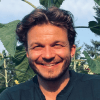
Sam Wright
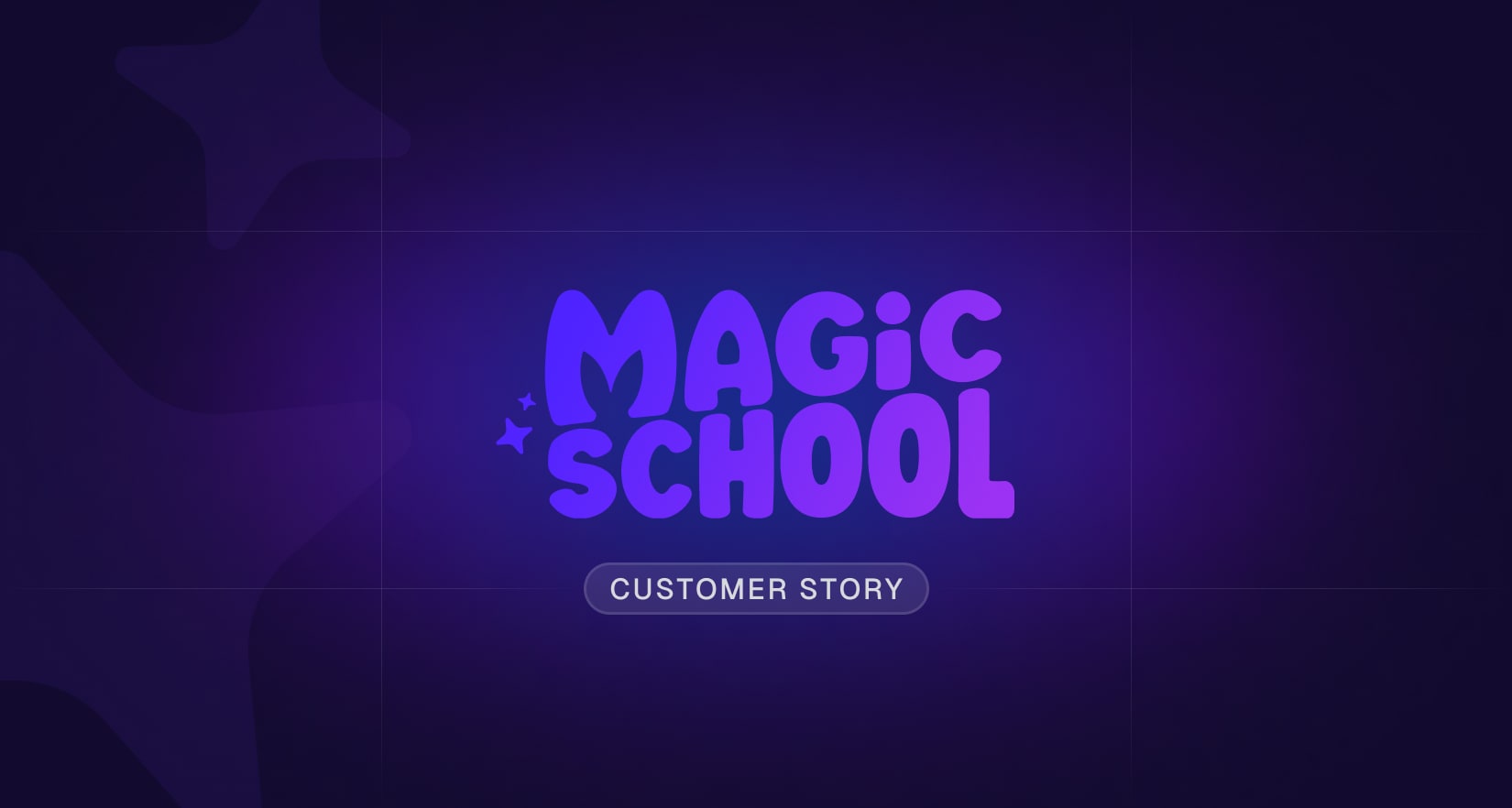
Customer story
How MagicSchool AI develops insights using Trigger.dev
Ben Duggan, Staff Software Engineer at MagicSchool AI, shares how they use Trigger.dev and Vercel's AI SDK to extract insights from millions of student interactions.
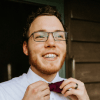
Ben Duggan
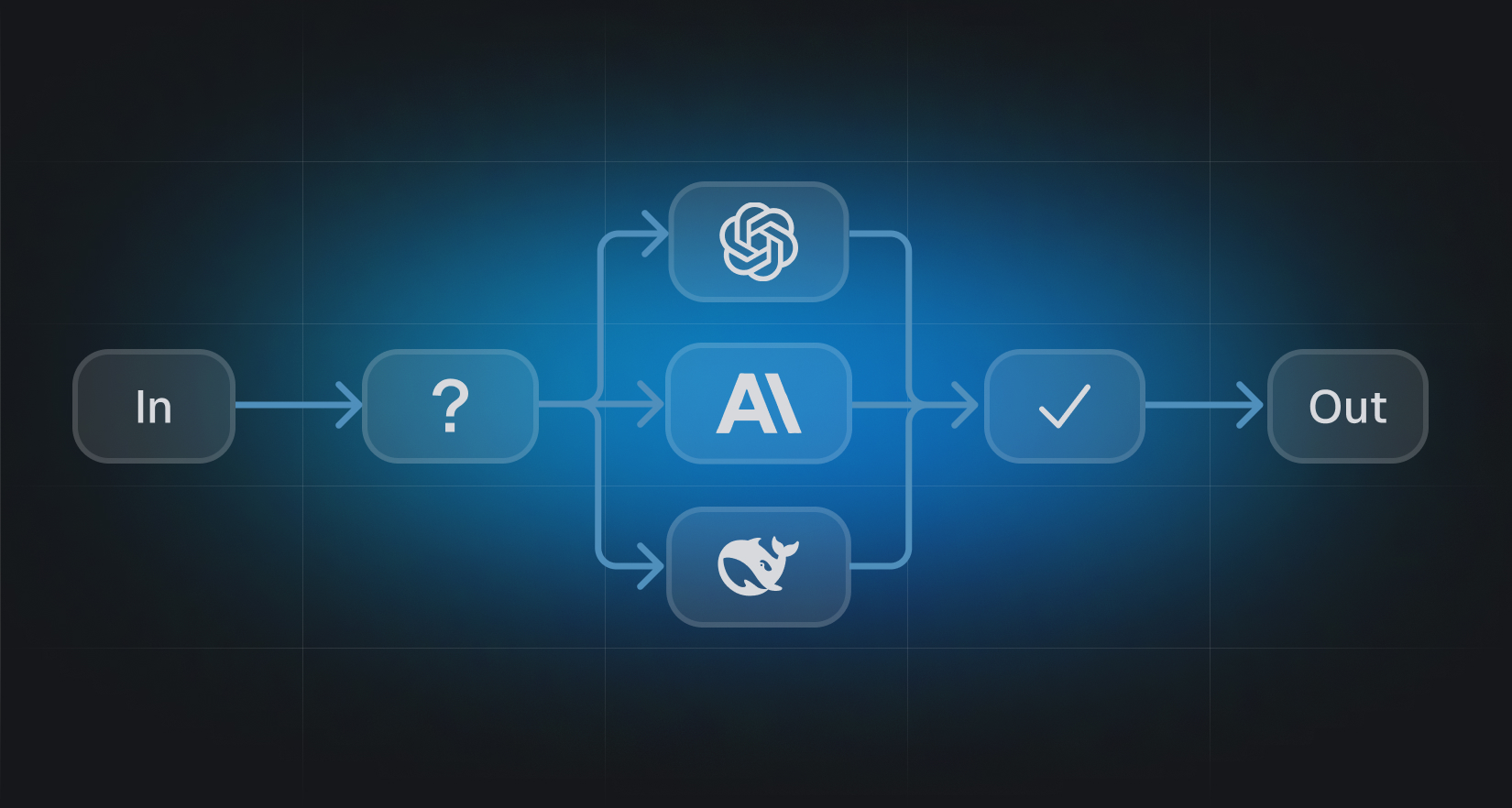
Article
Building effective AI agents with Trigger.dev
Learn how to build AI agents with Trigger.dev, and how they can help you build more resilient systems.
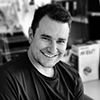
James Ritchie
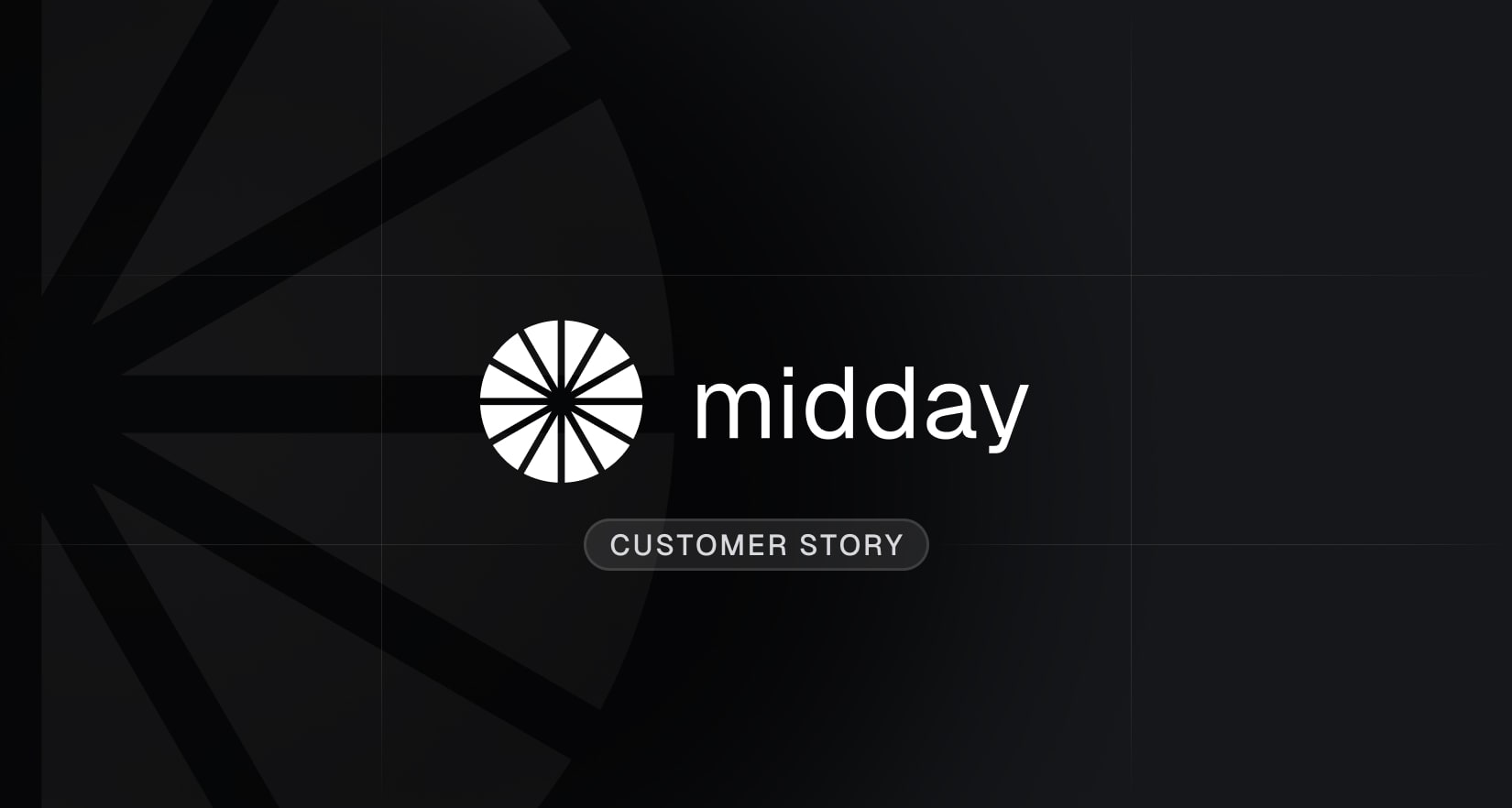
Customer story
Midday's Automated Bank Synchronization, powered by Trigger.dev
Pontus Abrahamsson, CEO and co-founder of Midday, deep dives into how they use Trigger.dev to reliably sync bank transactions for their 11,500+ customers.
Pontus Abrahamsson
![Everything we launched during launchweek[0]](/blog/launchweek0-recap/recap.jpg?1)
Launch week
Everything we launched during launchweek[0]
A recap of all the new features we announced during our first launch week.
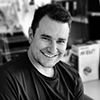
James Ritchie
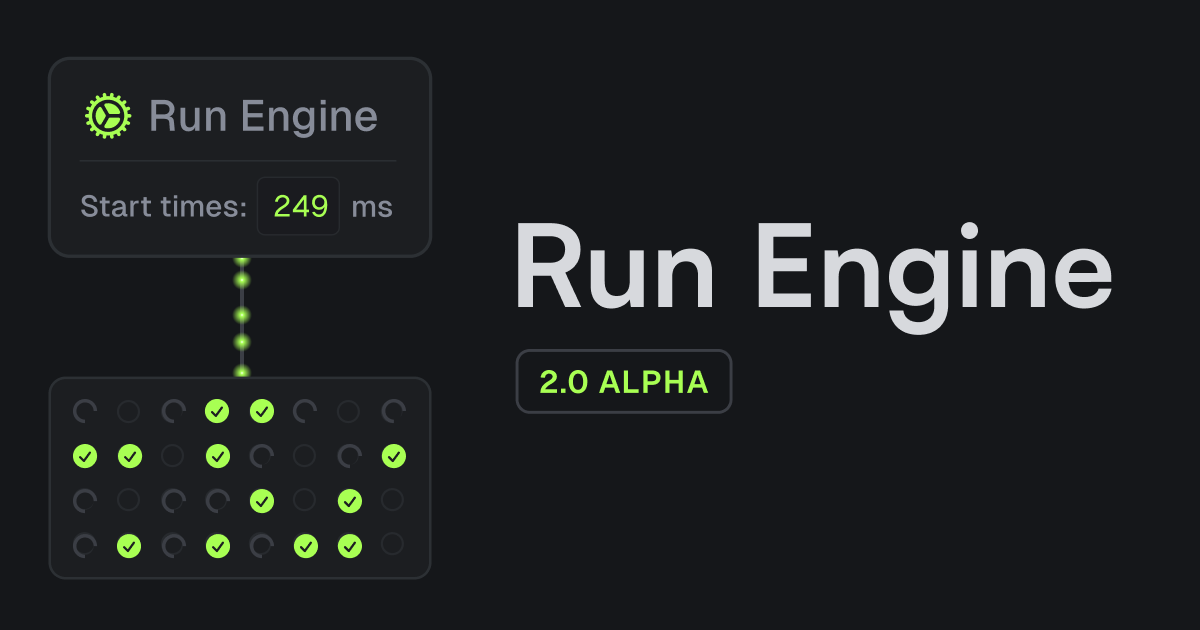
Launch week
Run Engine 2.0 (alpha)
Warm starts, multi-region workers, self-hosted workers, and new waitpoints.
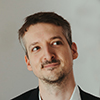
Matt Aitken
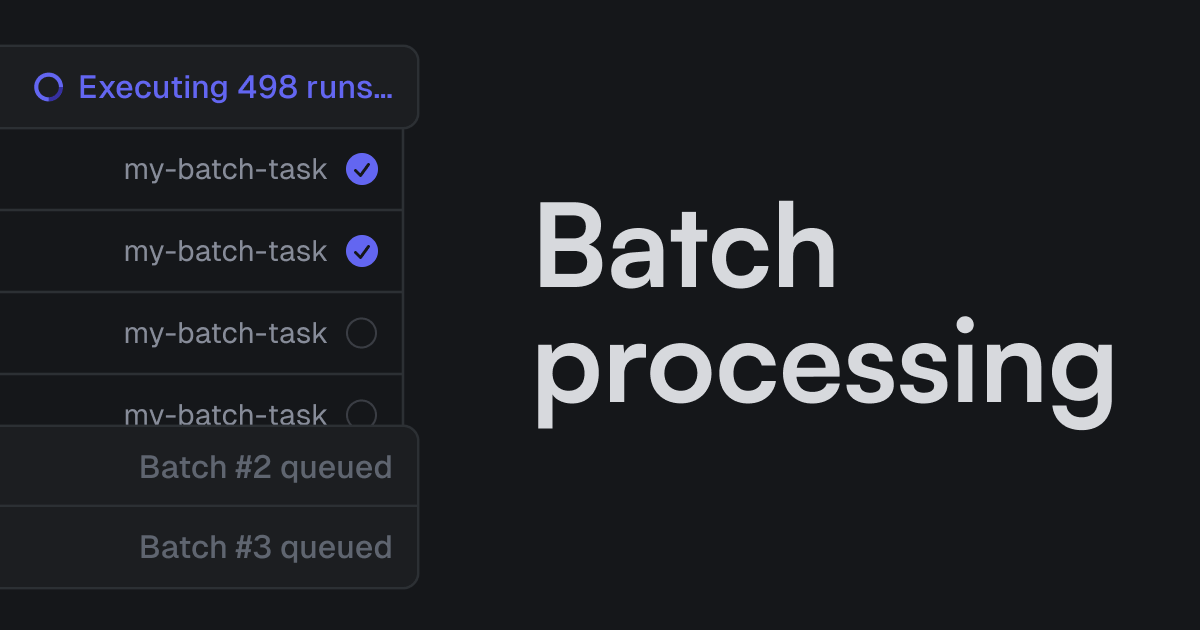
Launch week
Batch processing
A bunch of improvements to our batch processing system, including new limits and dashboard visibility.
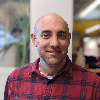
Eric Allam
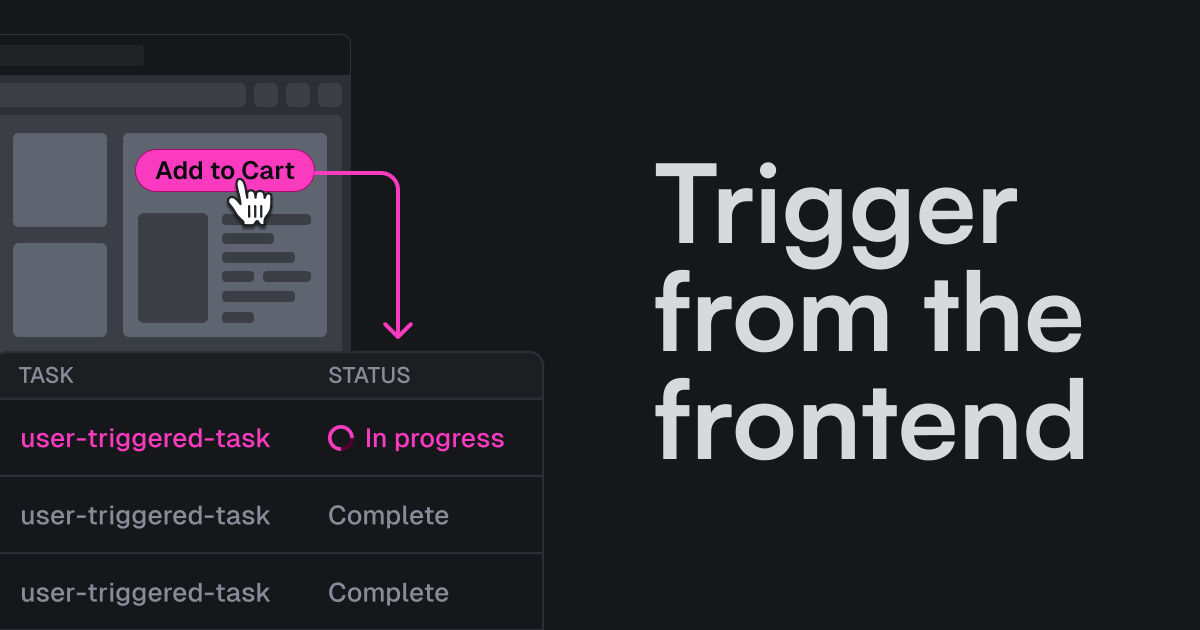
Launch week
Trigger from the frontend
Trigger.dev now supports triggering tasks from the frontend.
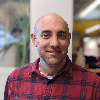
Eric Allam
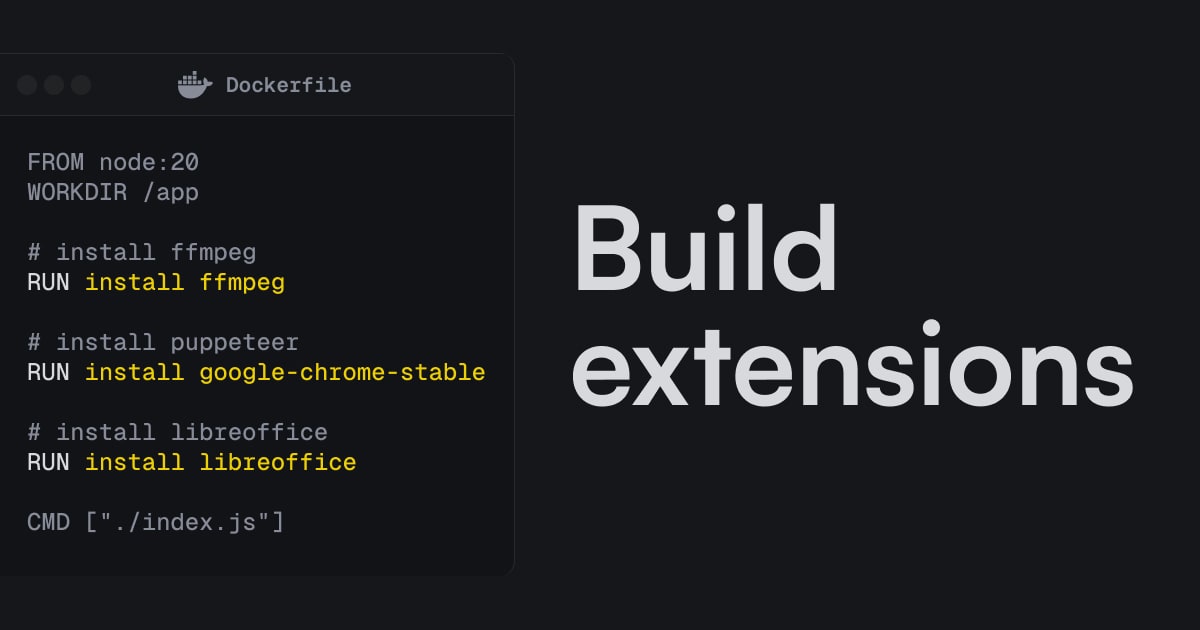
Launch week
Build extensions
Install system packages, like FFmpeg and Puppeteer, and modify the build process.
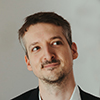
Matt Aitken
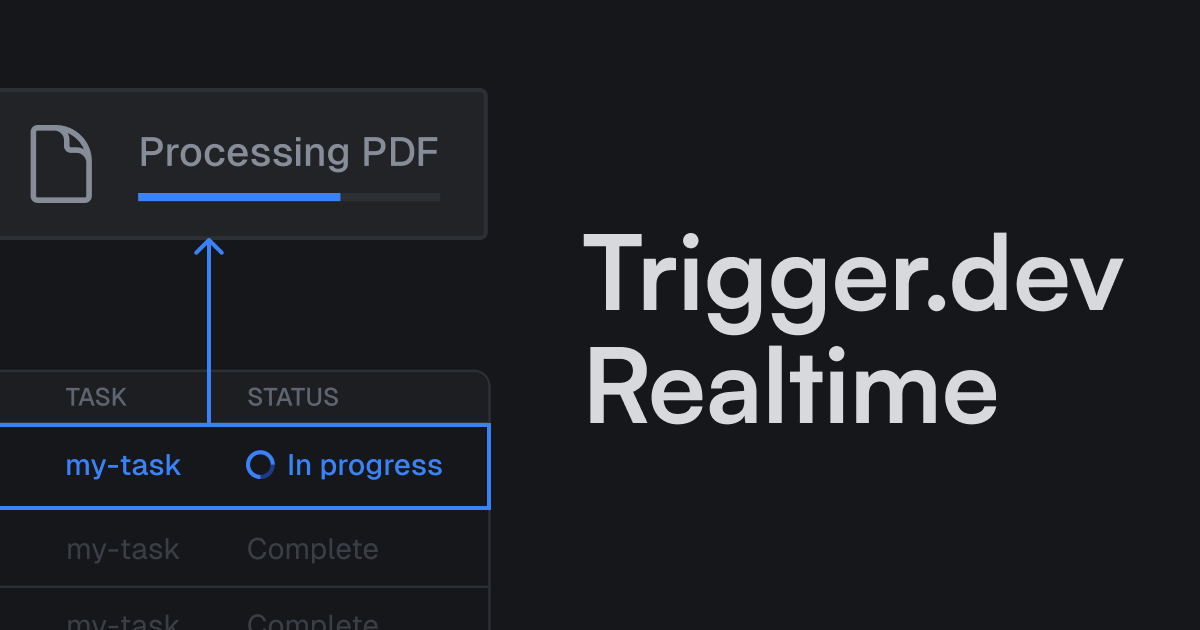
Launch week
Realtime goes GA
Keep your users updated with real-time task progress. Now with LLM streaming support and increased limits.
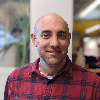
Eric Allam
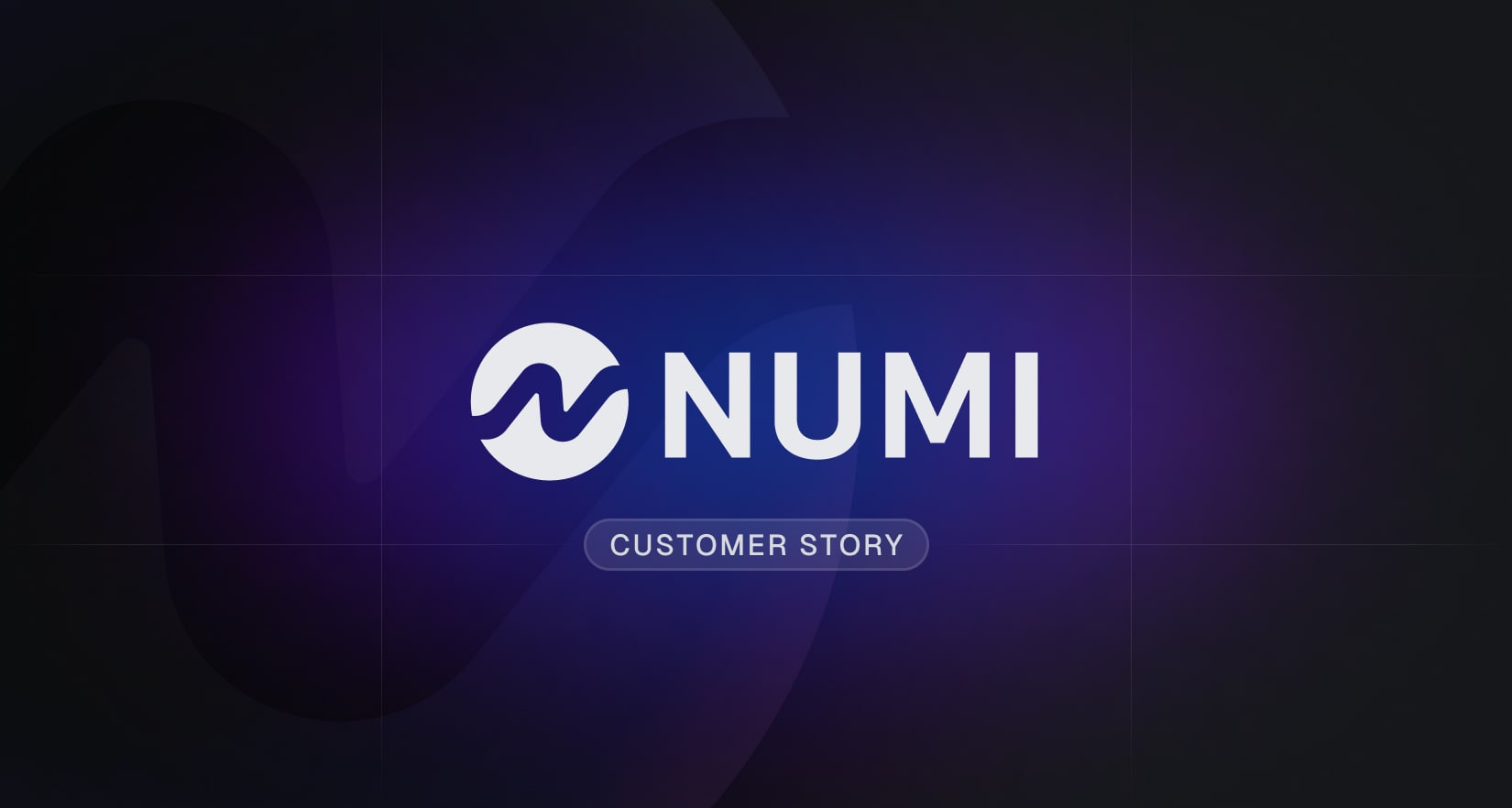
Customer story
How NUMI uses Trigger.dev to build resilient systems
Agree Ahmed, CEO of NUMI, shares his story of how they have re-architected their code to use Trigger.dev, and how it has changed the way they think about building complex distributed systems.
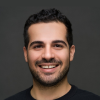
Agree Ahmed
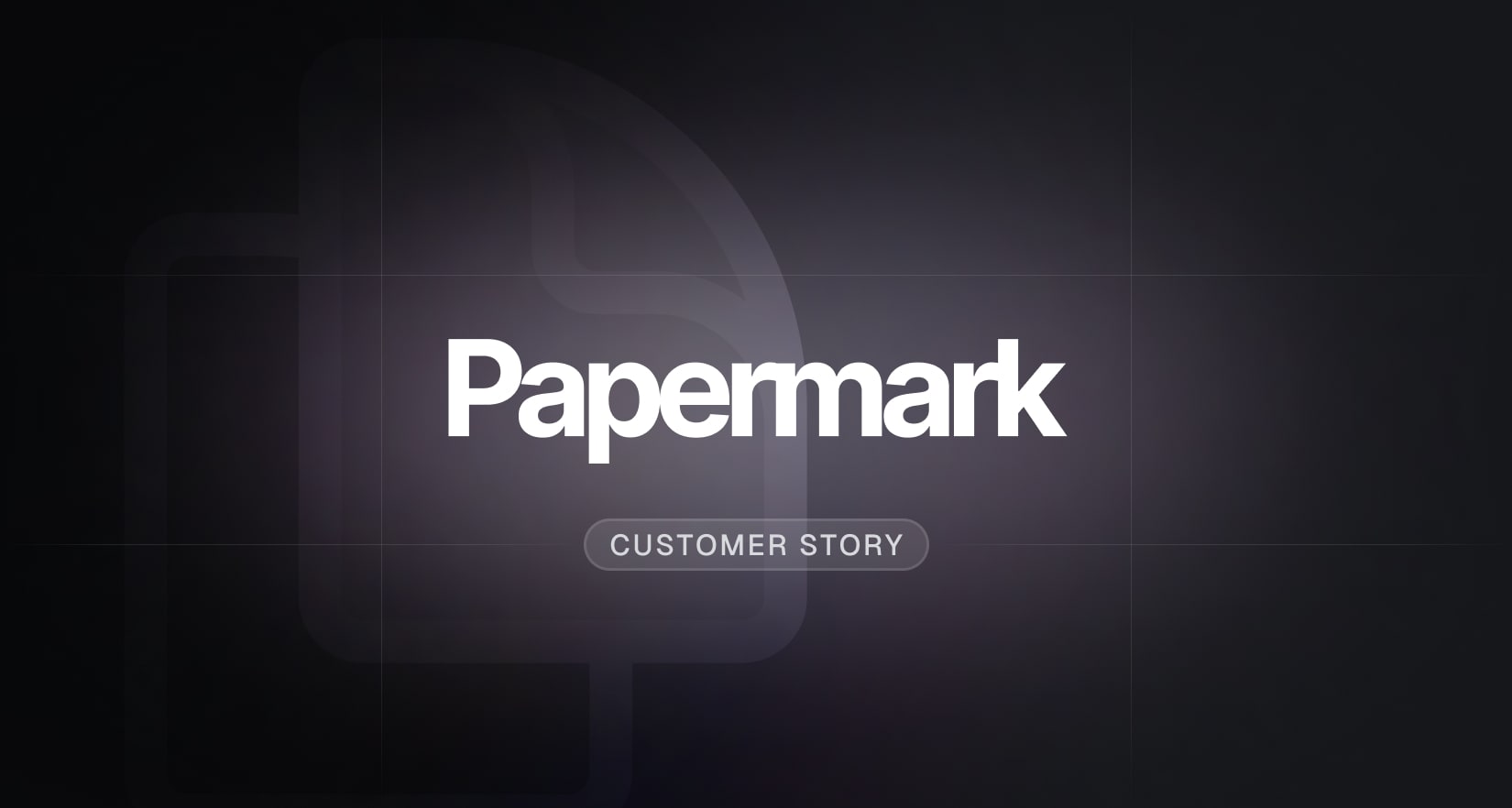
Customer story
Real-time PDF conversion with Trigger.dev: Papermark’s success story
Marc Seitz, co-founder of Papermark, runs through how they have used Trigger.dev to build a real-time PDF conversion service, with thousands of conversions successfully processed per month.
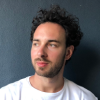
Marc Seitz
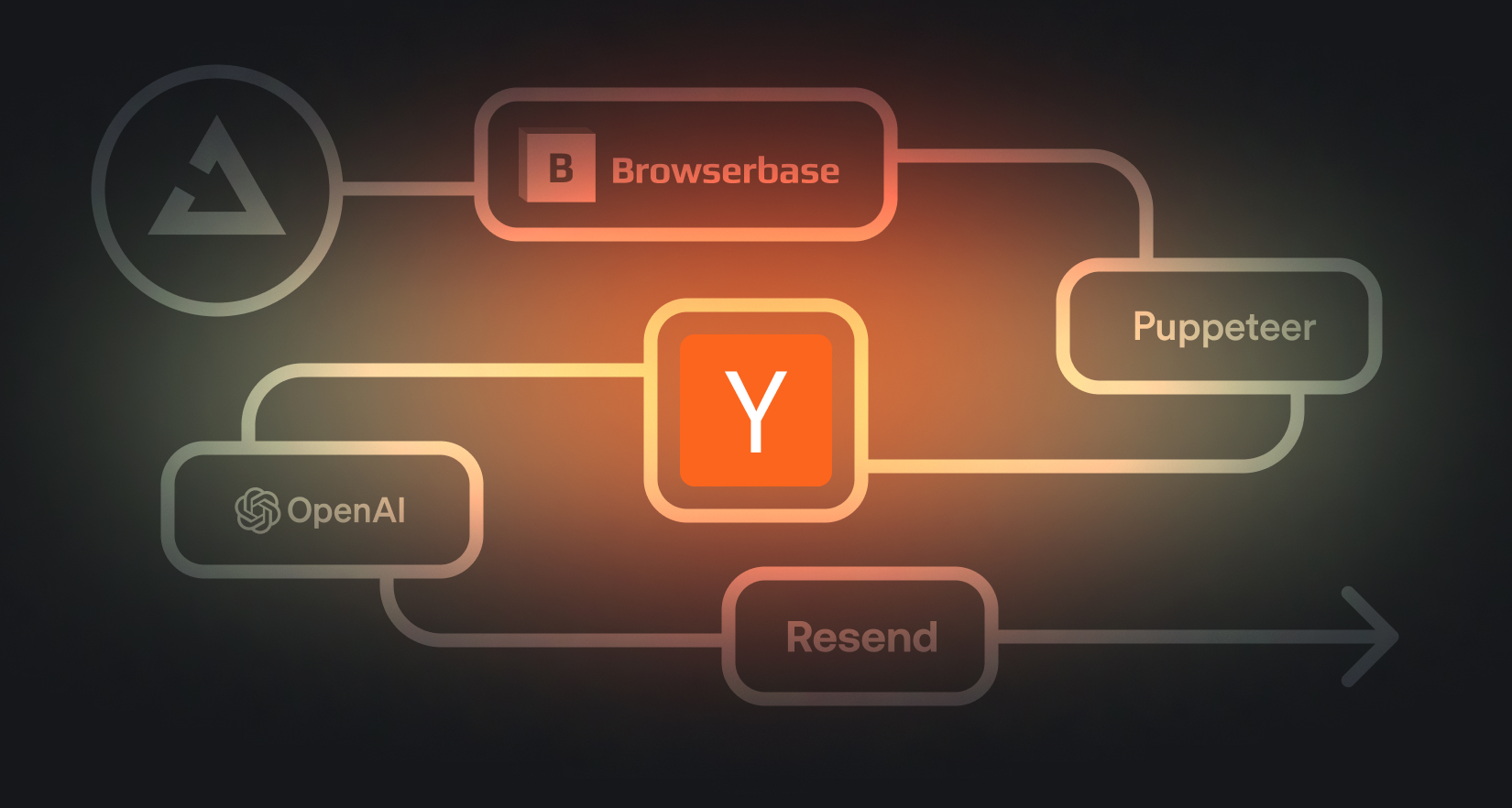
Article
How to scrape a website using Browserbase, Puppeteer, OpenAI and Trigger.dev
Learn how to scrape the top 3 articles from Hacker News and email yourself a summary every weekday at 9AM.
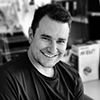
James Ritchie
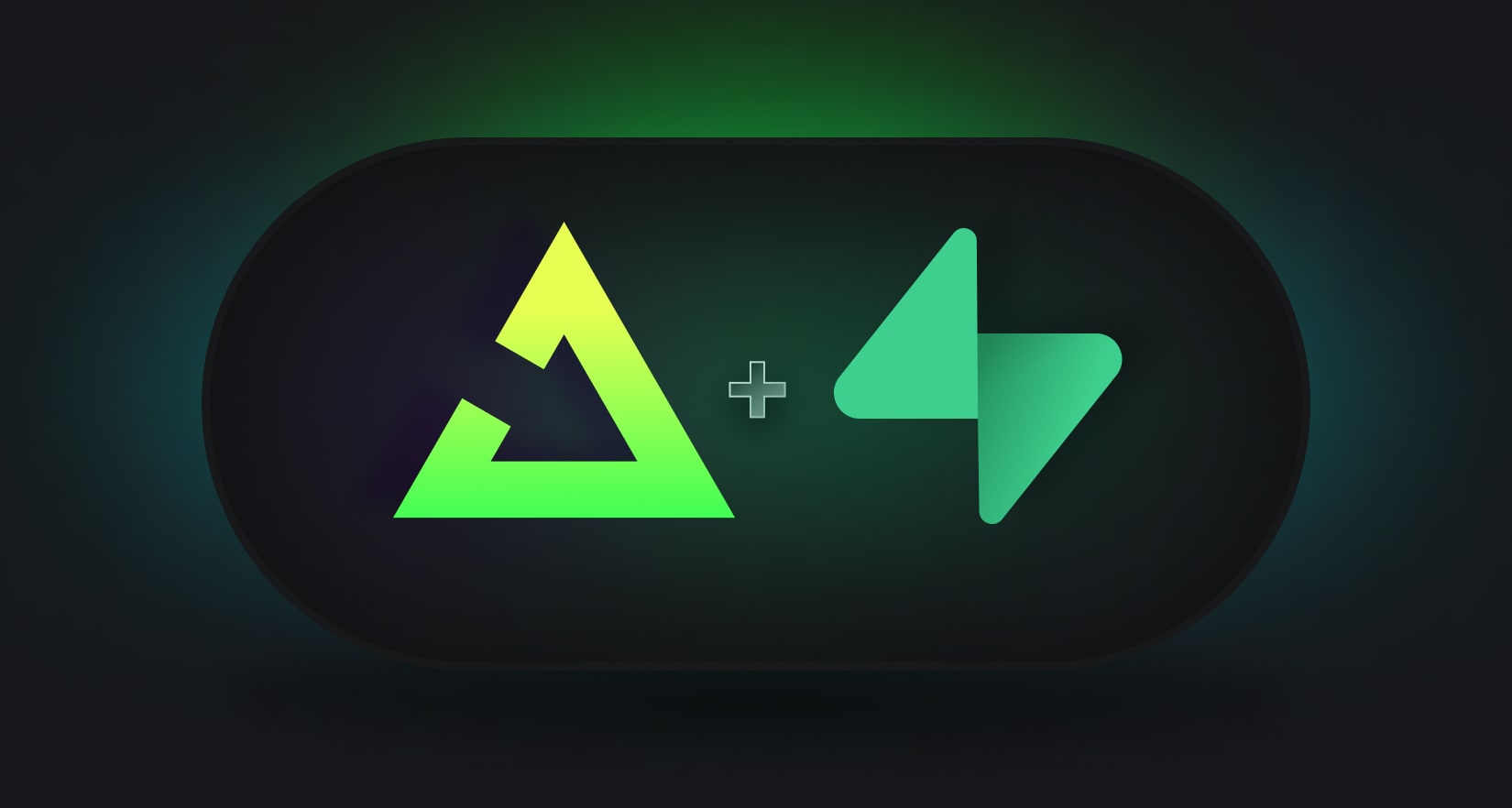
Article
Trigger anything from a database change using Supabase with Trigger.dev
Learn how to use Trigger.dev with Supabase to create event-driven workflows. We'll cover triggering tasks from Edge Functions, performing database operations, and building a video processing pipeline with AI transcription.
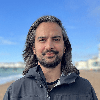
Dan Patel
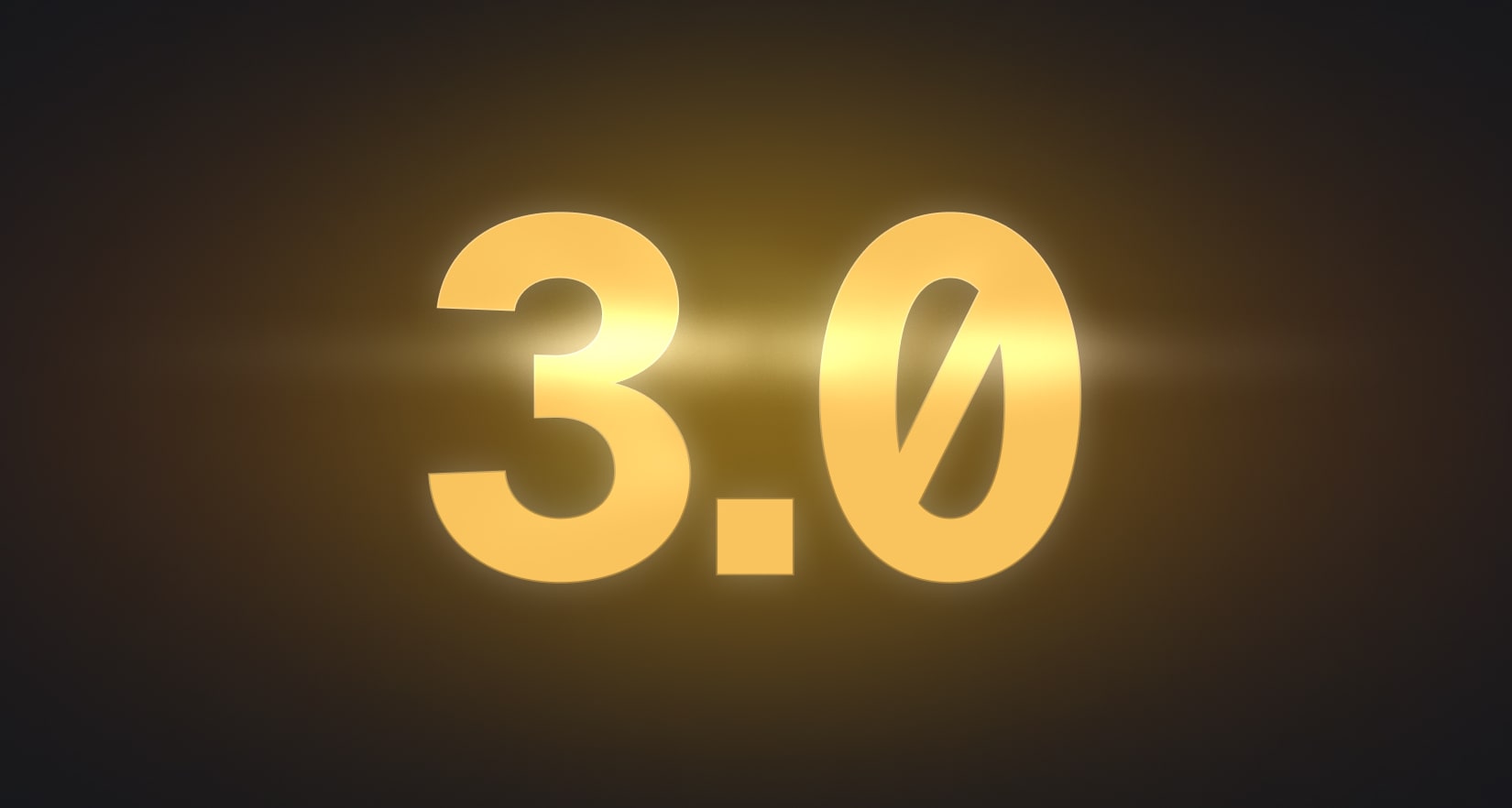
Article
From beta to 3.0: Trigger.dev v3 reaches GA
Trigger.dev v3 is now out of beta and at v3.0.0. This release includes a new CLI, a revamped build system, and more.
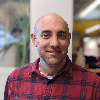
Eric Allam
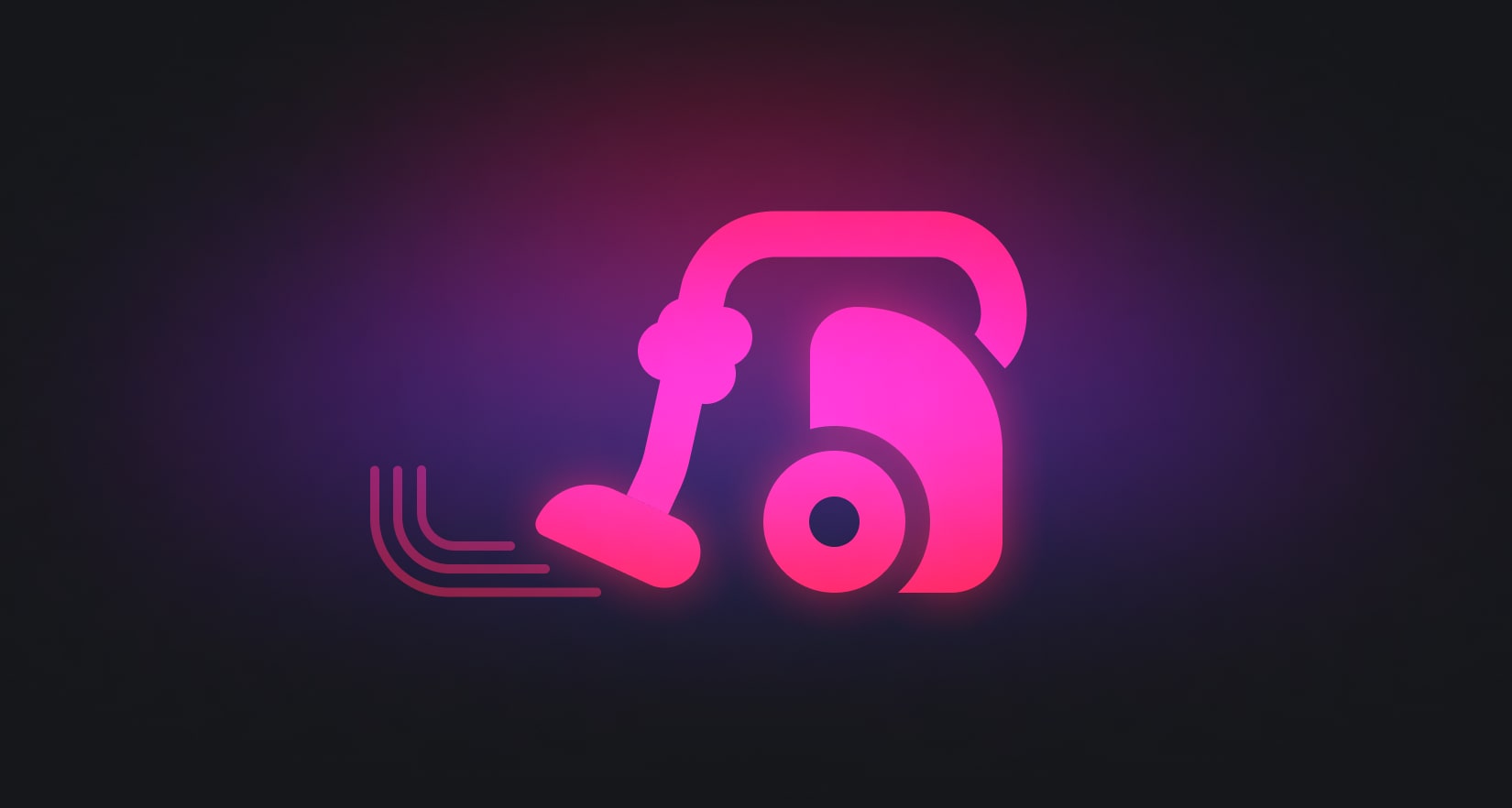
Article
How we stopped xmin horizon blocking Postgres vacuuming: a deepdive
We encountered an issue with xmin horizon blocking Postgres vacuuming, causing system slowdowns. Learn about our troubleshooting process, the solution we implemented, and our steps to prevent similar incidents in the future.
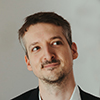
Matt Aitken
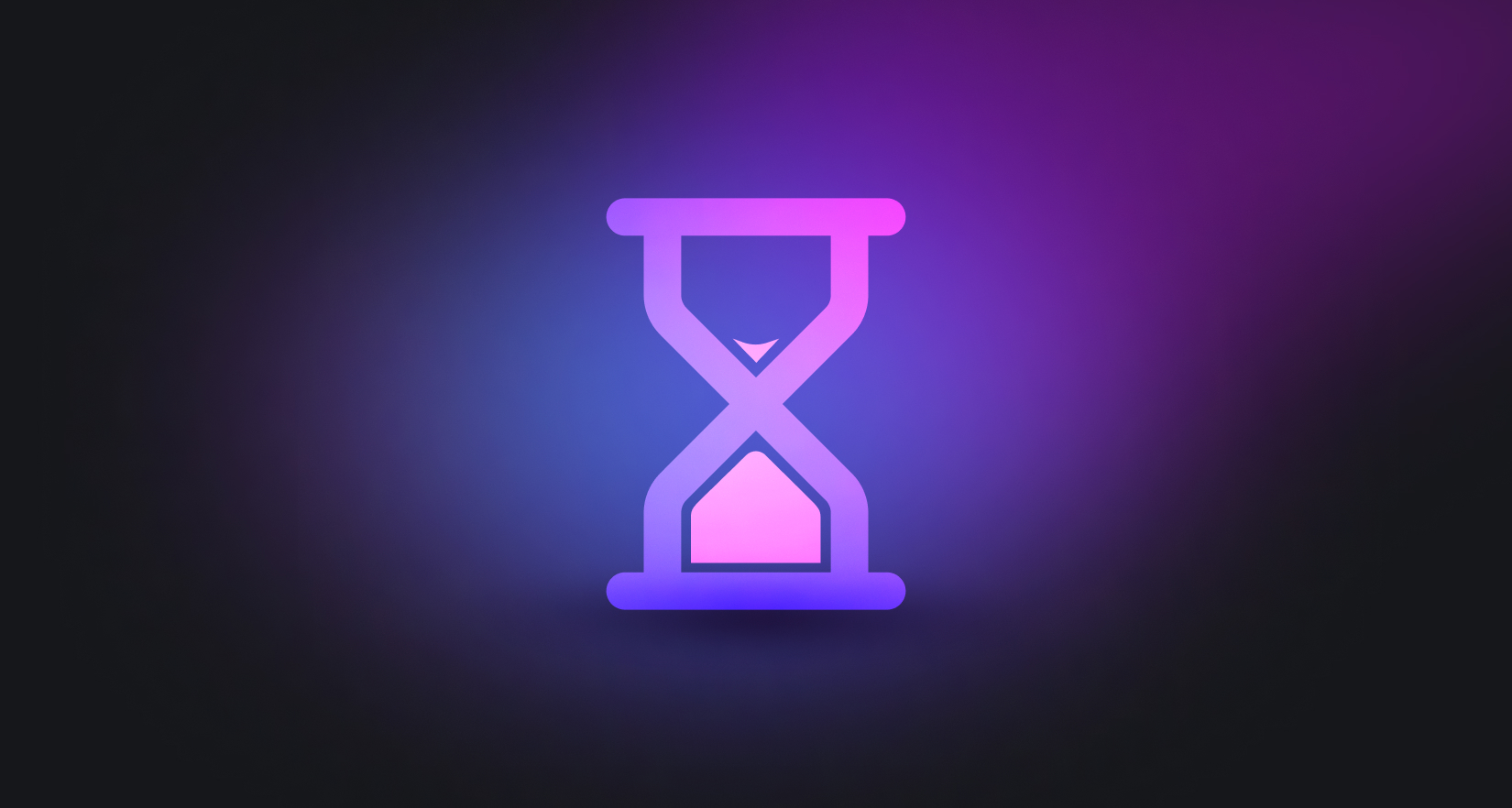
Announcement
Trigger.dev v2 end-of-life and how to upgrade to v3
Trigger.dev v2 will reach end-of-life on January 31st 2025. Here's what you need to know and how to migrate to v3.
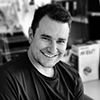
James Ritchie
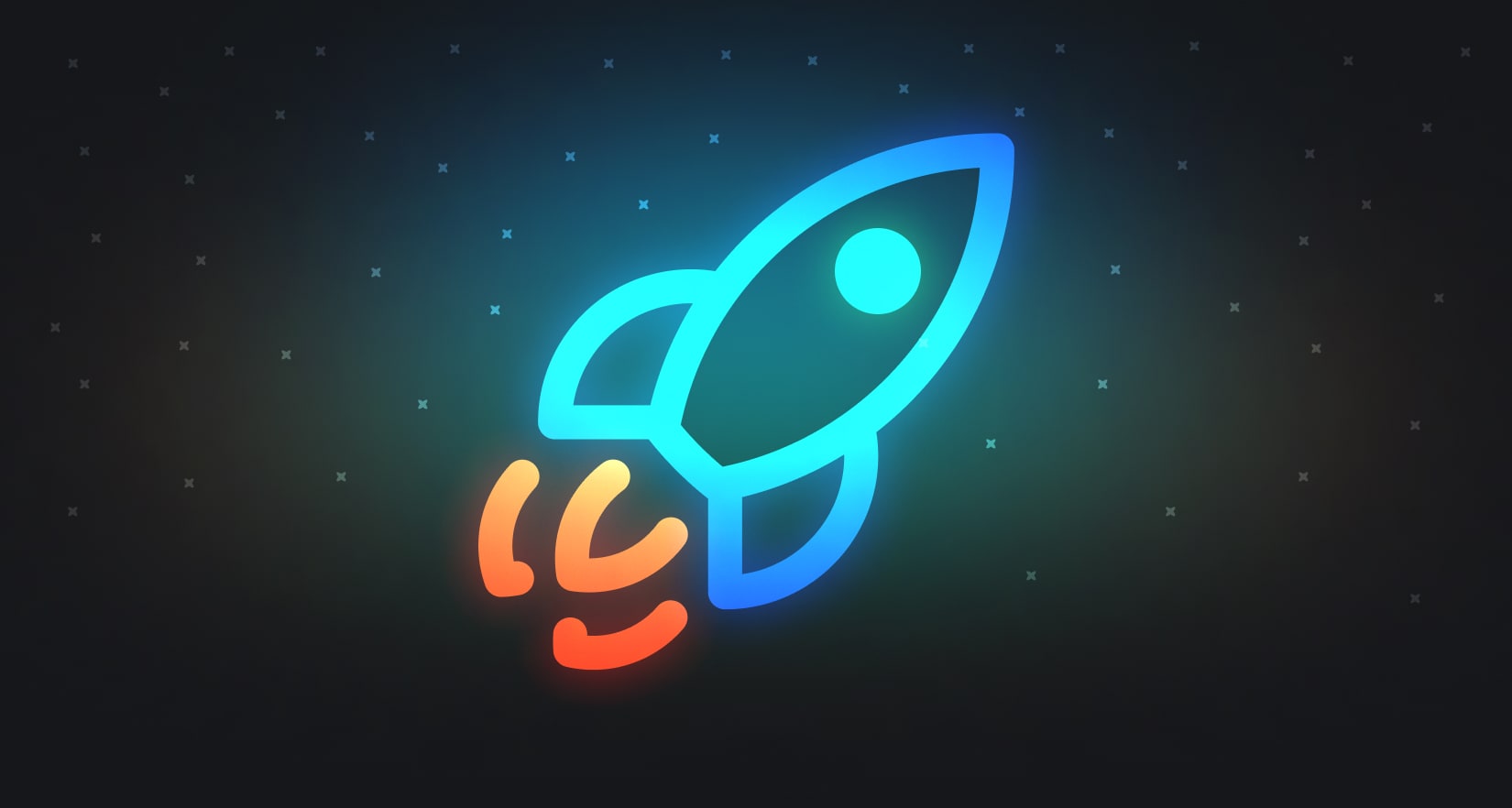
Announcement
Trigger.dev v3 is now open to everyone
Today, I'm excited to announce that Trigger.dev v3 is now open to everyone. No more waitlist!
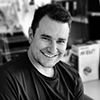
James Ritchie
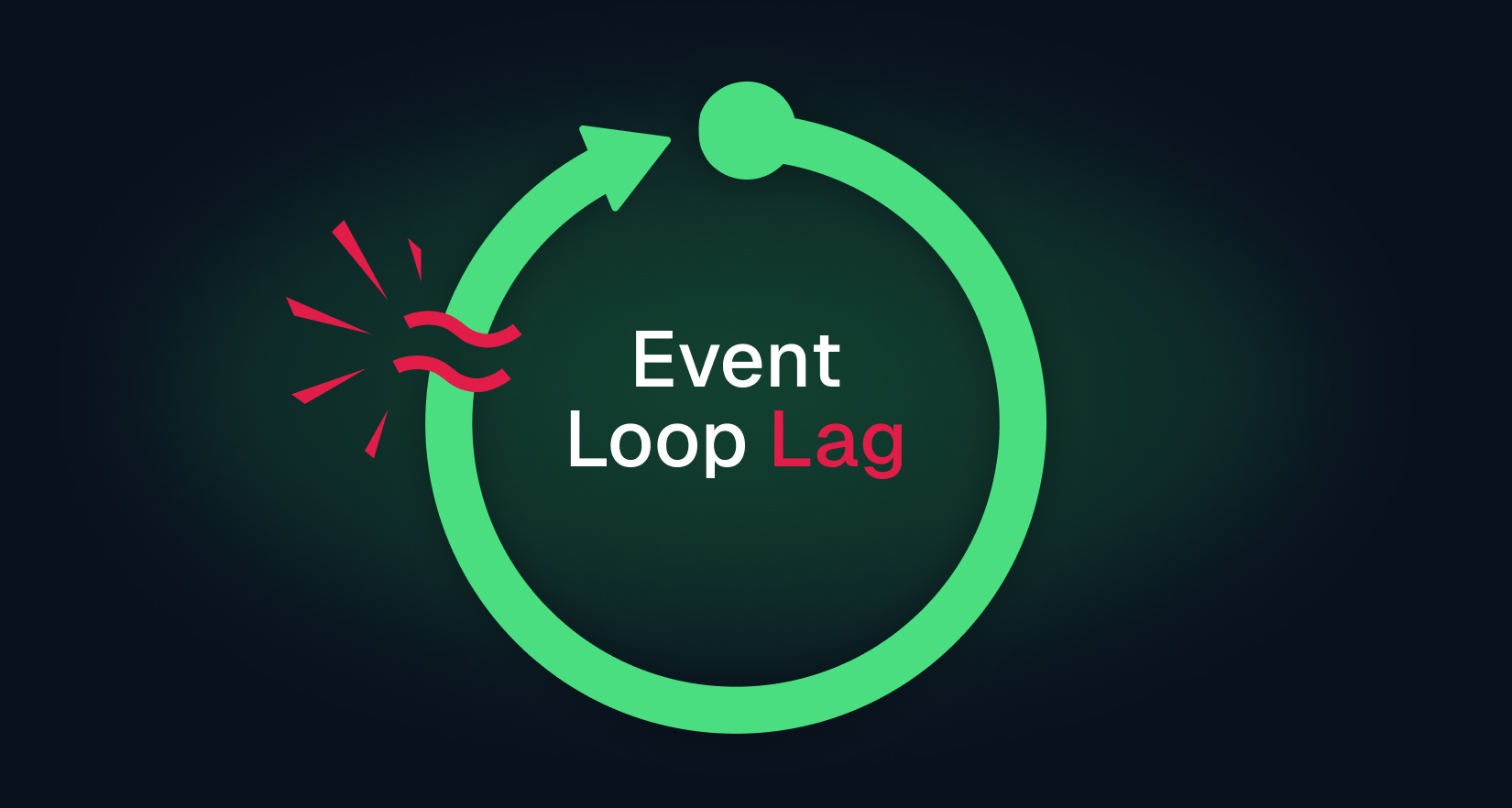
Article
How we tamed Node.js event loop lag: a deepdive
We recently experienced some service degradation and downtime due to event loop lag in our Node.js service. Here's how we diagnosed and fixed the issue.
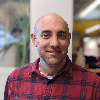
Eric Allam
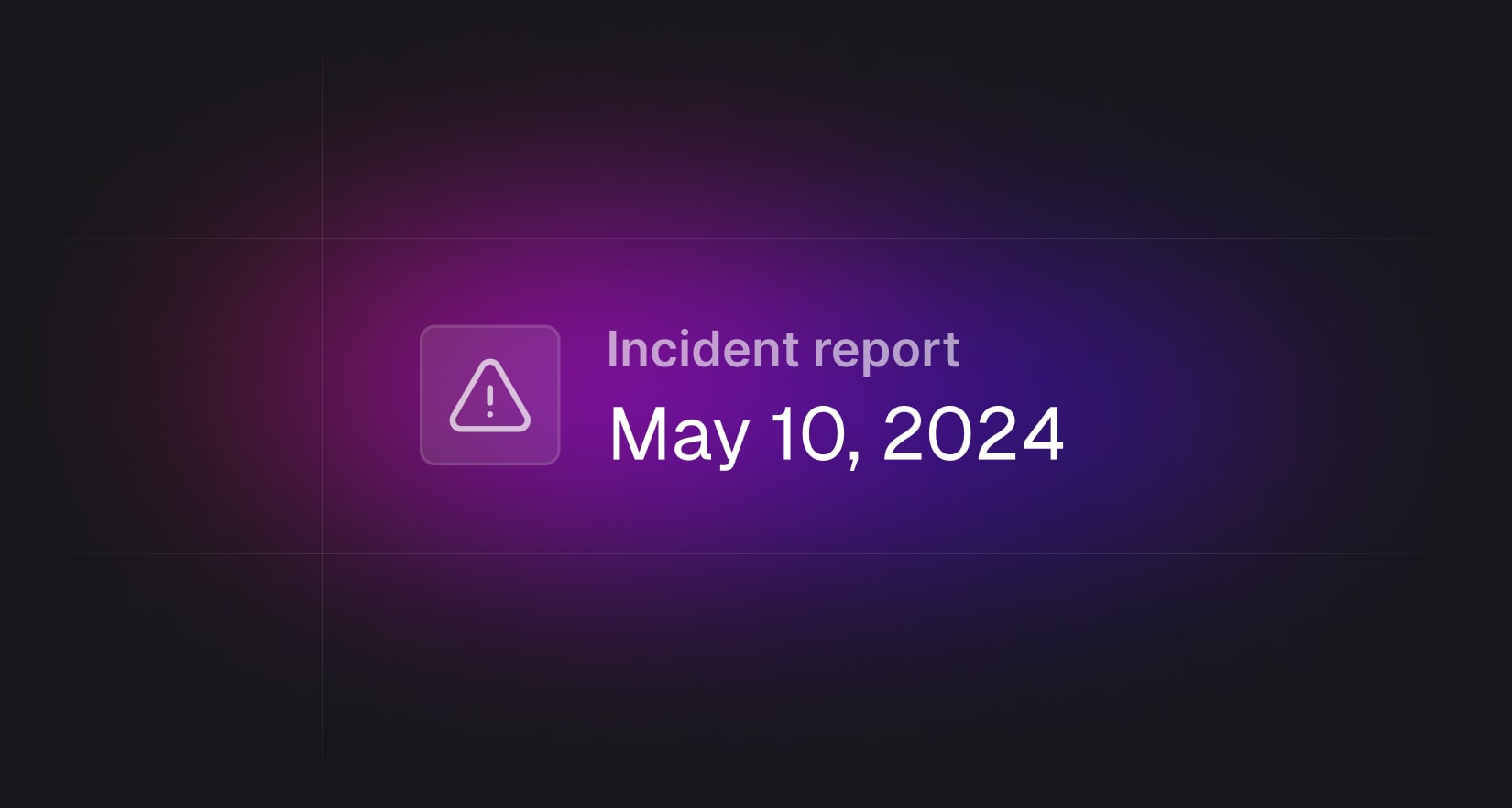
Incident
Incident report on May 10, 2024
There was a major incident which caused runs to process extremely slowly and the dashboard to be unavailable. Here's what happened and what we've changed to prevent it from happening again.
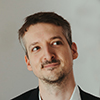
Matt Aitken
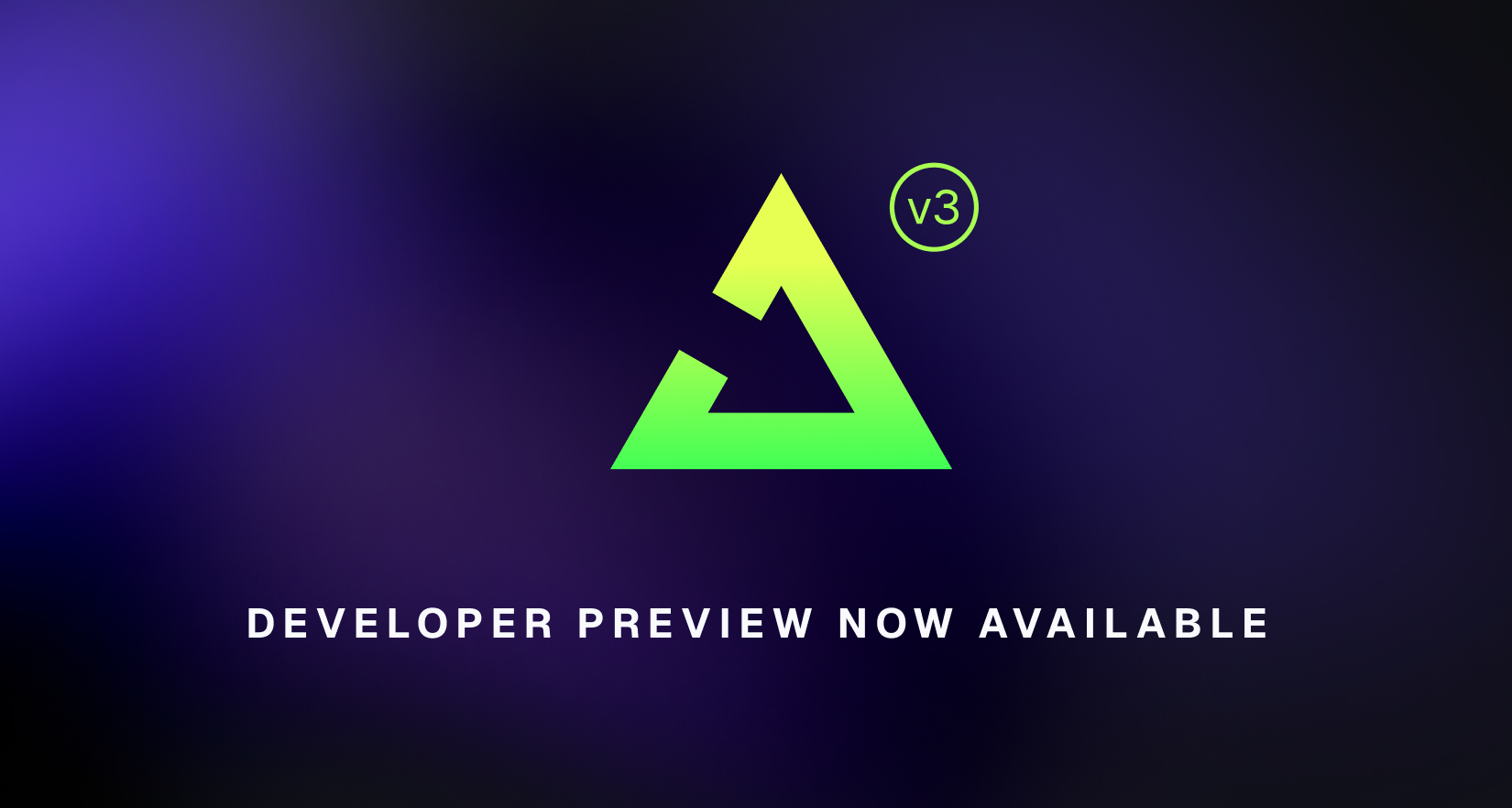
Announcement
Trigger.dev v3 Developer Preview is now available
Today you can get early access to v3. No timeouts, easy reliability and a new dashboard for finding and fixing bugs.
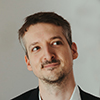
Matt Aitken
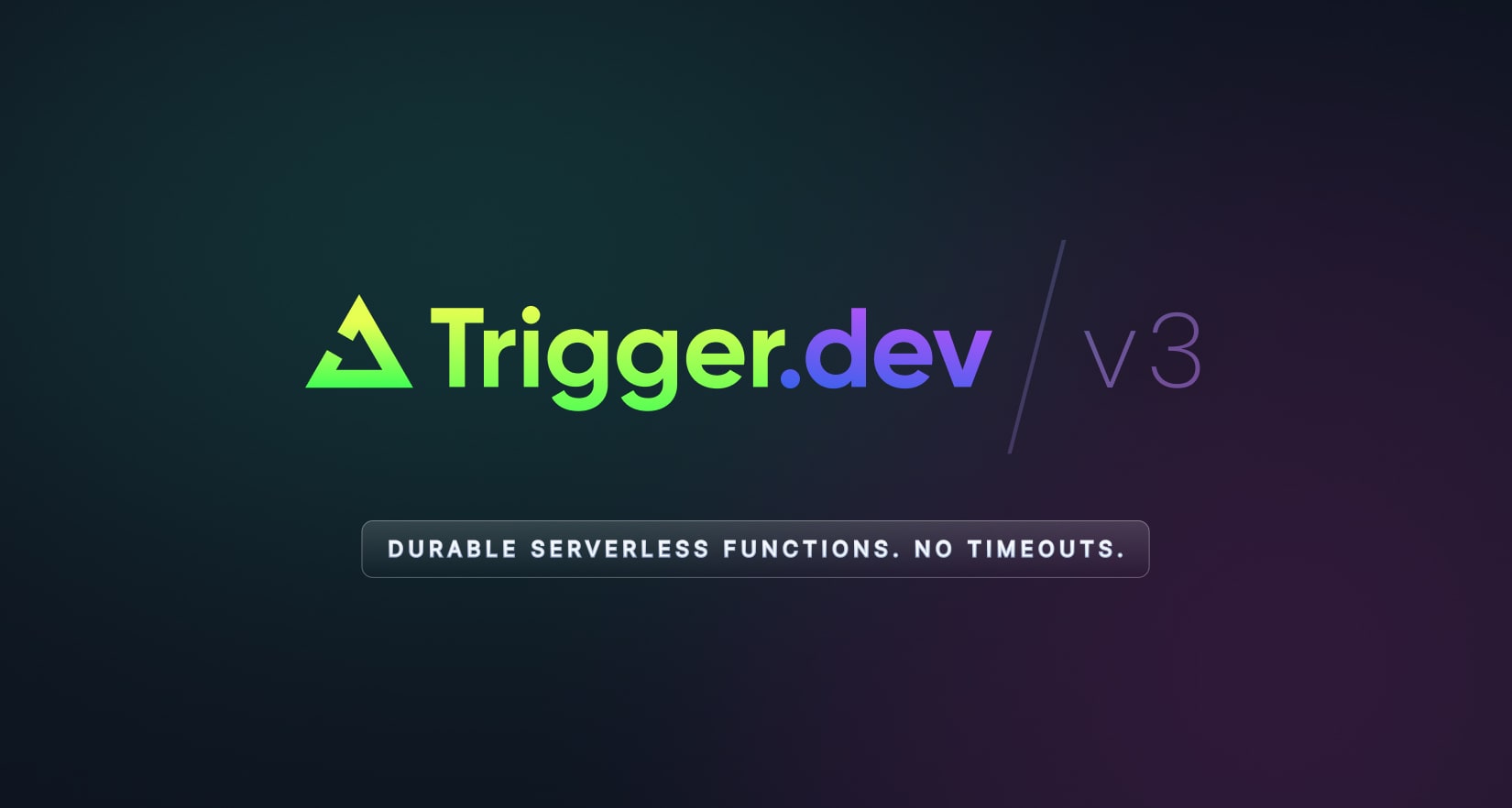
Announcement
Trigger.dev v3: Durable Serverless functions. No timeouts.
Write regular code and gvet durability with no timeouts. This means writing long-running tasks is far easier than before.
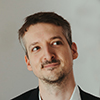
Matt Aitken
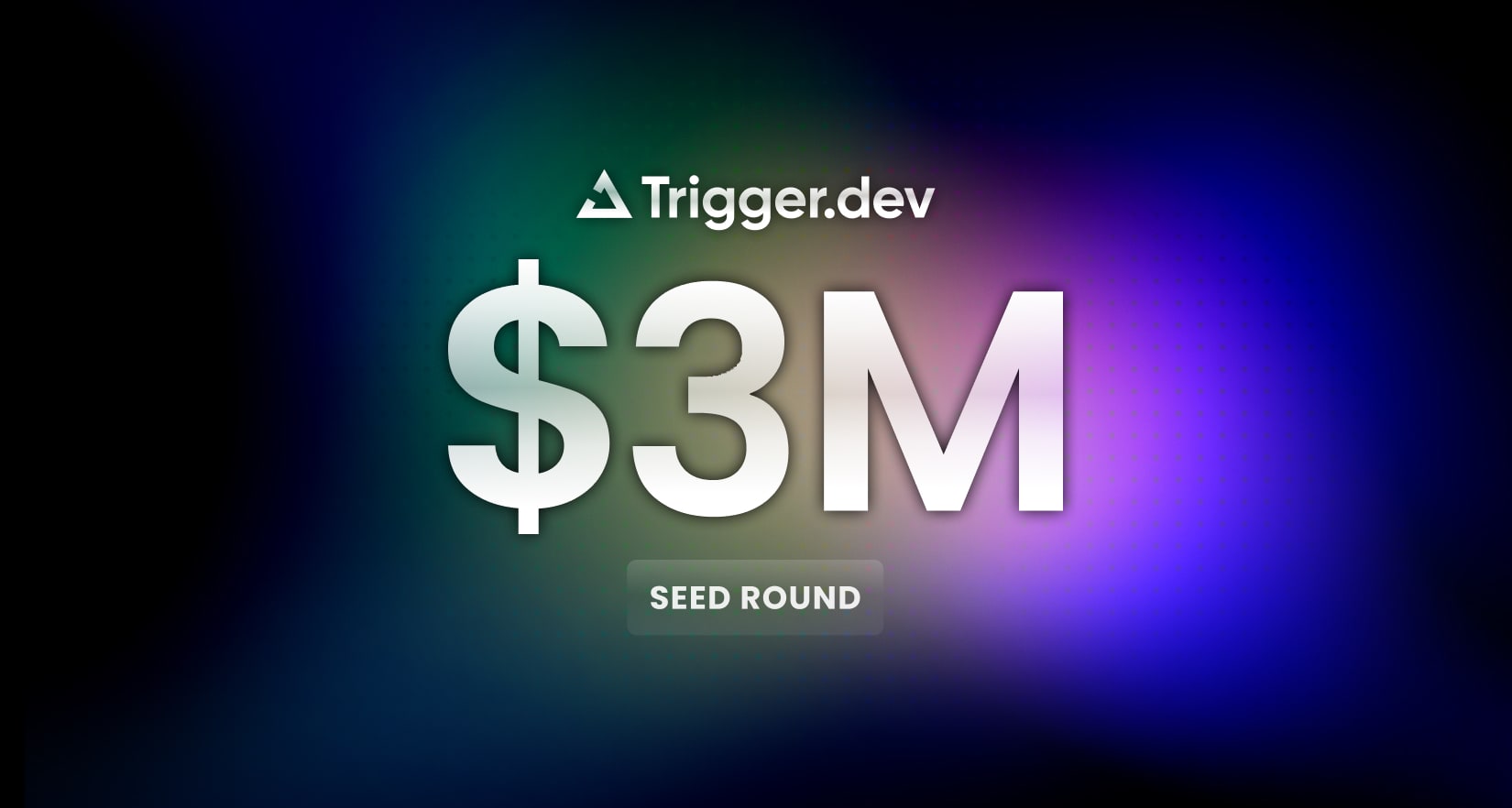
Announcement
Trigger.dev raises $3M
Trigger.dev, the open source background jobs framework, has today announced a $3M seed round.
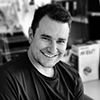
James Ritchie