Triggering tasks with webhooks in Next.js
Learn how to trigger a task from a webhook in a Next.js app.
Prerequisites
- A Next.js project, set up with Trigger.dev
- cURL installed on your local machine. This will be used to send a POST request to your webhook handler.
GitHub repo
View the project on GitHub
Click here to view the full code for this project in our examples repository on GitHub. You can fork it and use it as a starting point for your own project.
Adding the webhook handler
The webhook handler in this guide will be an API route.
This will be different depending on whether you are using the Next.js pages router or the app router.
Pages router: creating the webhook handler
Create a new file pages/api/webhook-handler.ts
or pages/api/webhook-hander.js
.
In your new file, add the following code:
This code will handle the webhook payload and trigger the ‘Hello World’ task.
App router: creating the webhook handler
Create a new file in the app/api/webhook-handler/route.ts
or app/api/webhook-handler/route.js
.
In your new file, add the following code:
This code will handle the webhook payload and trigger the ‘Hello World’ task.
Triggering the task locally
Now that you have your webhook handler set up, you can trigger the ‘Hello World’ task from it. We will do this locally using cURL.
Run your Next.js app and the Trigger.dev dev server
First, run your Next.js app.
Then, open up a second terminal window and start the Trigger.dev dev server:
Trigger the webhook with some dummy data
To send a POST request to your webhook handler, open up a terminal window on your local machine and run the following command:
If http://localhost:3000
isn’t the URL of your locally running Next.js app, replace the URL in
the below command with that URL instead.
This will send a POST request to your webhook handler, with a JSON payload.
Check the task ran successfully
After running the command, you should see a successful dev run and a 200 response in your terminals.
If you now go to your Trigger.dev dashboard, you should also see a successful run for the ‘Hello World’ task, with the payload you sent, in this case; {"name": "John Doe", "age": "87"}
.
Learn more about Next.js and Trigger.dev
Walk-through guides from development to deployment
Next.js - setup guide
Learn how to setup Trigger.dev with Next.js, using either the pages or app router.
Next.js - triggering tasks using webhooks
Learn how to create a webhook handler for incoming webhooks in a Next.js app, and trigger a task from it.
Task examples
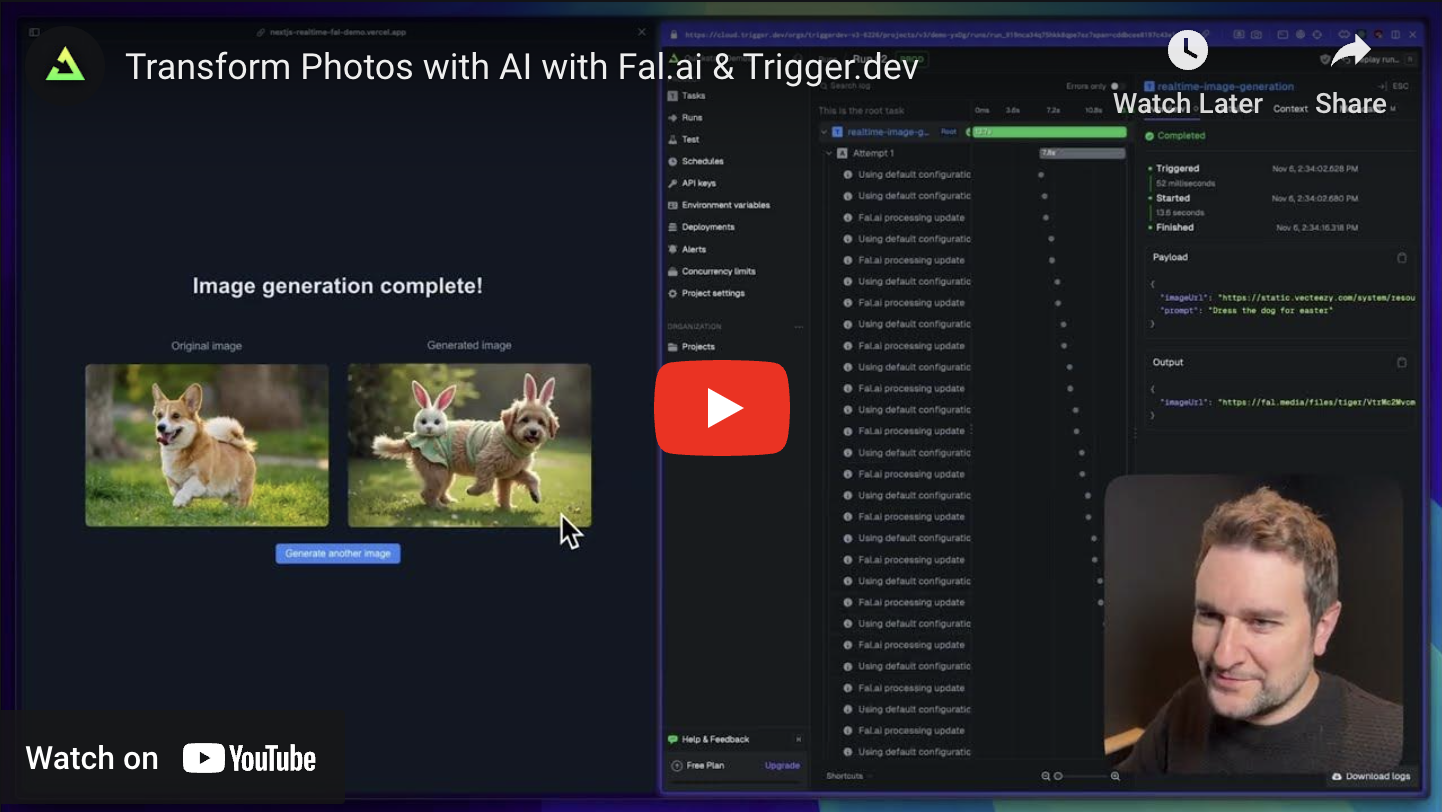
Fal.ai with Realtime in Next.js
Generate an image from a prompt using Fal.ai and Trigger.dev Realtime.
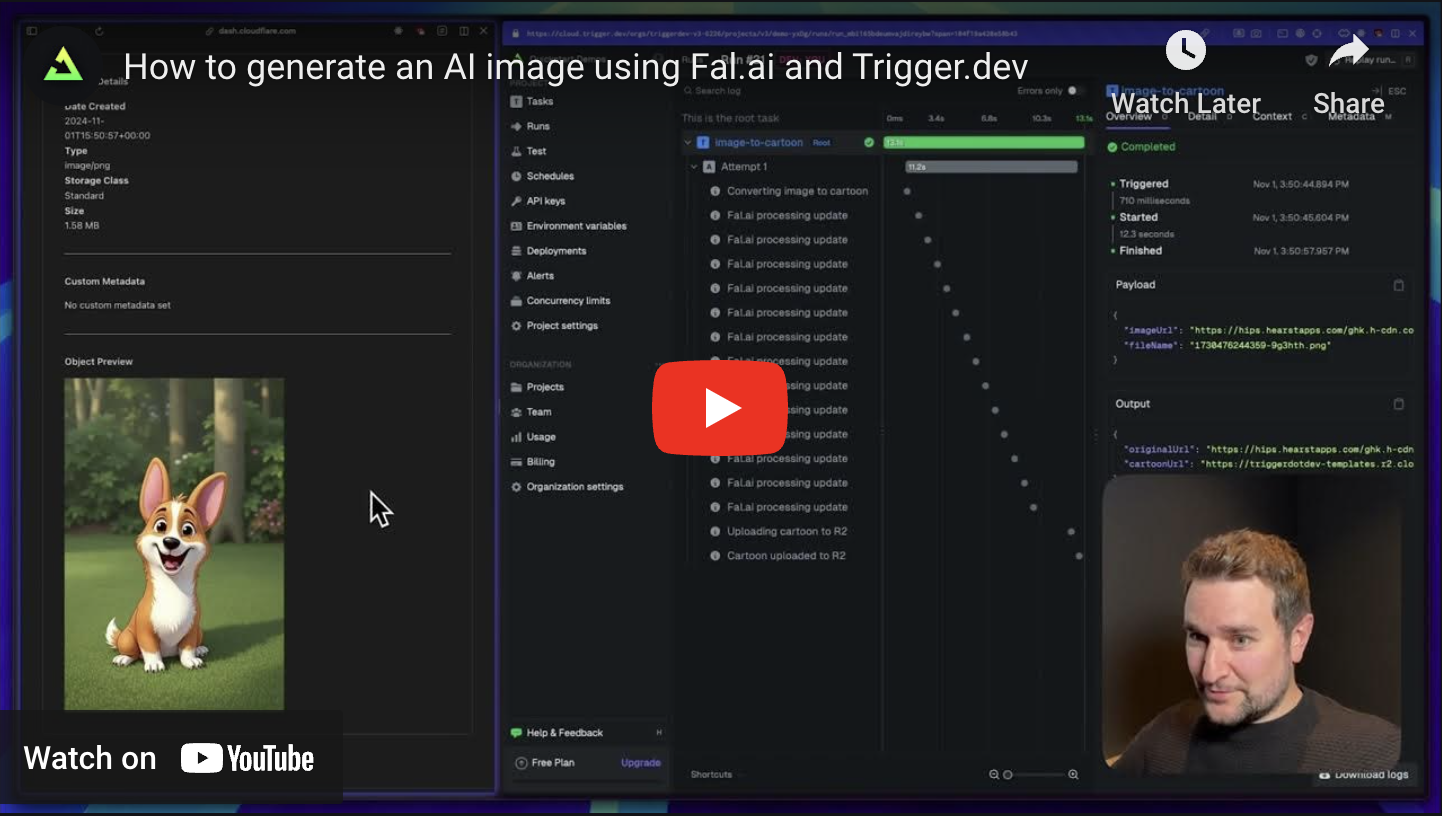
Generate a cartoon using Fal.ai in Next.js
Convert an image to a cartoon using Fal.ai.
Vercel sync environment variables
Learn how to automatically sync environment variables from your Vercel projects to Trigger.dev.
Vercel AI SDK
Learn how to use the Vercel AI SDK, which is a simple way to use AI models from different providers, including OpenAI, Anthropic, Amazon Bedrock, Groq, Perplexity etc.