Changelog #34
HTTP endpoints: trigger jobs from any webhooks
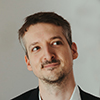
CEO, Trigger.dev
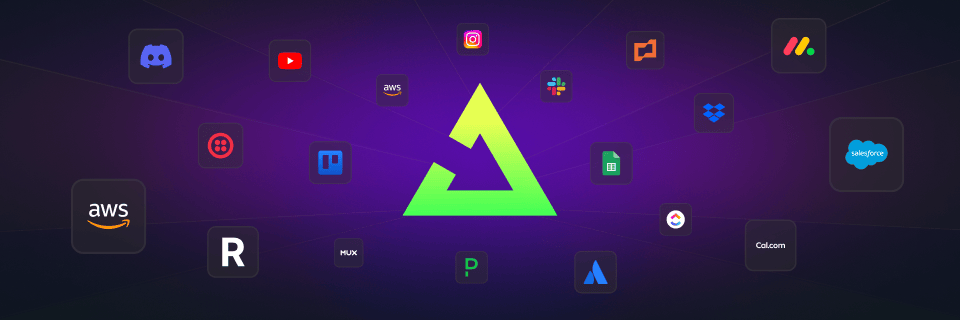
Often you want to trigger your Jobs from events that happen in other APIs. This is where webhooks come in.
Now you can easily subscribe to any APIs that support webhooks, without needing to use a Trigger.dev Integration. This should unlock you to create far more Jobs than was previously possible.
How to create an HTTP endpoint
We want to send a Slack message when one of our cal.com meetings is cancelled. To do this we need to create an HTTP endpoint that cal.com can send a webhook to.
_15//create an HTTP endpoint_15const caldotcom = client.defineHttpEndpoint({_15 id: "cal.com",_15 source: "cal.com",_15 icon: "caldotcom",_15 verify: async (request) => {_15 //this helper function makes verifying most webhooks easy_15 return await verifyRequestSignature({_15 request,_15 headerName: "X-Cal-Signature-256",_15 secret: process.env.CALDOTCOM_SECRET!,_15 algorithm: "sha256",_15 });_15 },_15});
Getting the URL and secret from the Trigger.dev dashboard
There's a new section in the sidebar: "HTTP endpoints".
From there you can select the HTTP endpoint you just created and get the URL and secret. In this case, it's cal.com.
Each environment has a different Webhook URL so you can control which environment you want to trigger Jobs for.
Setting up the webhook in cal.com
In cal.com you can navigate to "Settings/Webhooks/New" to create a new webhook.
Enter the URL and secret from the Trigger.dev dashboard and select the events you want to trigger Jobs for.
We could only select "Booking cancelled" but we're going to select all the events so we can reuse this webhook for more than just a single trigger.
Using HTTP endpoints to create Triggers
Then we can use that HTTP endpoint to create multiple Triggers for your Jobs. They can have different filters, using the data from the webhook.
_24client.defineJob({_24 id: "http-caldotcom",_24 name: "HTTP Cal.com",_24 version: "1.0.0",_24 enabled: true,_24 //create a Trigger from the HTTP endpoint above. The filter is optional._24 trigger: caldotcom.onRequest({_24 filter: { body: { triggerEvent: ["BOOKING_CANCELLED"] } },_24 }),_24 run: async (request, io, ctx) => {_24 //note that when using HTTP endpoints, the first parameter is the request_24 //you need to get the body, usually it will be json so you do:_24 const body = await request.json();_24_24 //this prints out "Matt Aitken cancelled their meeting"_24 await io.logger.info(_24 `${body.payload.attendees_24 .map((a) => a.name)_24 .join(", ")} cancelled their meeting ${new Date(_24 body.payload.startTime_24 )}`_24 );_24 },_24});
See our HTTP endpoint docs for more info. Upgrade to the latest version of the SDK to start using this feature.
How to update
The Trigger.dev Cloud is now running v2.2.4
. If you are self-hosting you can upgrade by pinning to the v2.2.4
tag.
The trigger.dev/*
packages are now at v2.2.5
. You can update using the following command:
_10npx @trigger.dev/cli@latest update