Changelog #39
Task Library & more tasks
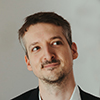
CEO, Trigger.dev
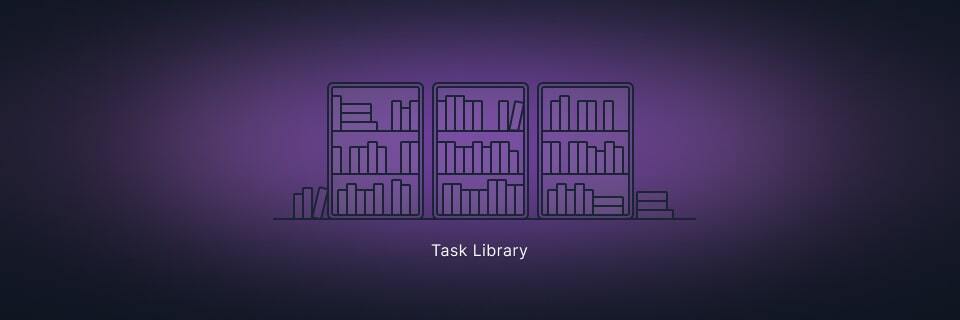
Task Library
We now have a dedicated docs page called Task Library where you can easily find and learn about built-in tasks to use in your jobs, like waitForEvent() or backgroundFetch():
New Tasks
We also have a few new tasks that we've added to the library:
io.backgroundPoll()
This task is similar to backgroundFetch
, but instead of waiting for a single request to complete, it will poll a URL until it returns a certain value:
_12const result = await io.backgroundPoll<{ foo: string }>("🔃", {_12 url: "https://example.com/api/endpoint",_12 interval: 10, // every 10 seconds_12 timeout: 300, // stop polling after 5 minutes_12 responseFilter: {_12 // stop polling once this filter matches_12 status: [200],_12 body: {_12 status: ["SUCCESS"],_12 },_12 },_12});
We even display each poll request in the run dashboard:
See our reference docs to learn more.
io.sendEvents()
io.sendEvents() allows you to send multiple events at a time:
_14await io.sendEvents("send-events", [_14 {_14 name: "new.user",_14 payload: {_14 userId: "u_12345",_14 },_14 },_14 {_14 name: "new.user",_14 payload: {_14 userId: "u_67890",_14 },_14 },_14]);
See our reference docs to learn more.
io.random()
io.random()
is identical to Math.random()
when called without options but ensures your random numbers are not regenerated on resume or retry. It will return a pseudo-random floating-point number between optional min (default: 0, inclusive) and max (default: 1, exclusive). Can optionally round to the nearest integer.
How to update
The trigger.dev/*
packages are now at v2.2.6
. You can update using the following command:
_10npx @trigger.dev/cli@latest update