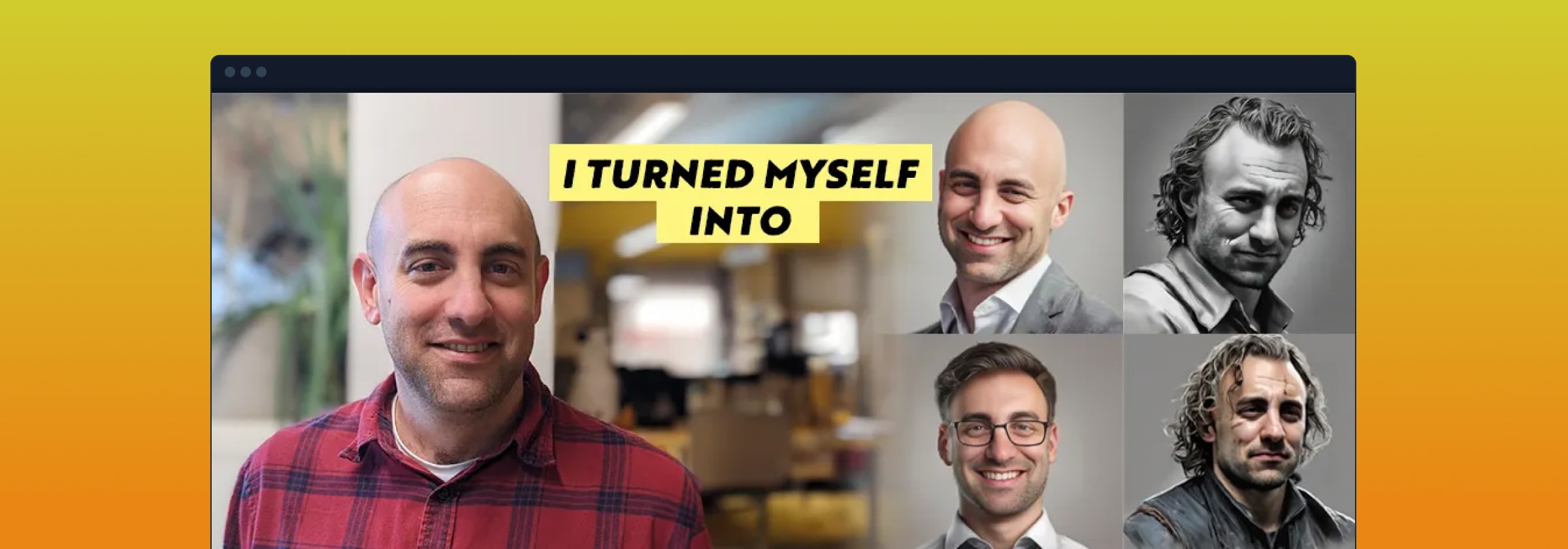
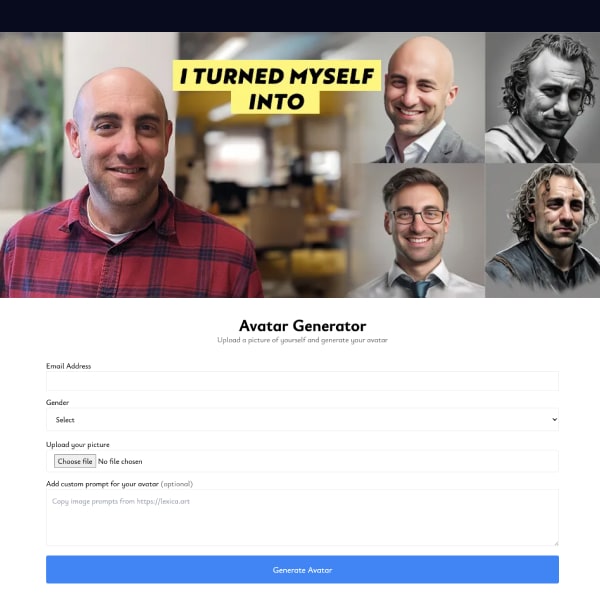
Turn yourself into a Superhero using AI
Trigger: eventTrigger
Build a web application that allows users to generate AI images of themselves based on the prompt provided.
Framework:
Repository:
/avatar-generator
Categories:
AI
Overview
A web application that allows users to generate AI images of themselves based on the prompt provided.
Features
- Seamlessly uploading images using Next.js, (hosted on Vercel)
- Generating AI images using our Replicate integration.
- Sending email using our Resend integration.
- Using React hooks to show the live status of the Job in the UI
Preview
How Trigger.dev is used in this project:
Job 1: Generating the image and swapping the face with Replicate
Replicate is a web platform that allows users to run models at scale in the cloud.
This job generates and swaps the faces using AI models on Replicate.
_36import { z } from "zod";_36_36client.defineJob({_36 id: "generate-avatar",_36 name: "Generate Avatar",_36 //👇🏻 integrates Replicate_36 integrations: { replicate },_36 version: "0.0.1",_36 trigger: eventTrigger({_36 name: "generate.avatar",_36 schema: z.object({_36 image: z.string(),_36 email: z.string(),_36 gender: z.string(),_36 userPrompt: z.string().nullable(),_36 }),_36 }),_36 run: async (payload, io, ctx) => {_36 const { email, image, gender, userPrompt } = payload;_36_36 await io.logger.info("Avatar generation started!", { image });_36_36 const imageGenerated = await io.replicate.run("create-model", {_36 identifier: process.env.STABILITY_AI_URI,_36 input: {_36 prompt: `${_36 userPrompt_36 ? userPrompt_36 : `A professional ${gender} portrait suitable for a social media avatar. Please ensure the image is appropriate for all audiences.`_36 }`,_36 },_36 });_36_36 await io.logger.info(JSON.stringify(imageGenerated));_36 },_36});
The code snippet above gets the data URI for the AI-generated and user's image and sends both images to the AI model, which returns the URL of the swapped image.
Job 2: Send the image to the user with Resend
Resend is an email API that enables you to send texts, attachments, and email templates easily.
This job sends the completed images to the user via email using Resend.
_33client.defineJob({_33 id: "generate-avatar",_33 name: "Generate Avatar",_33 // ---👇🏻 integrates Resend ---_33 integrations: { resend },_33 version: "0.0.1",_33 trigger: eventTrigger({_33 name: "generate.avatar",_33 schema: z.object({_33 image: z.object({ filepath: z.string() }),_33 email: z.string(),_33 gender: z.string(),_33 userPrompt: z.string().nullable(),_33 }),_33 }),_33 run: async (payload, io, ctx) => {_33 const { email, image, gender, userPrompt } = payload;_33 //👇🏻 -- After swapping the images, add the code snipped below --_33 await io.logger.info("Swapped image: ", { swappedImage });_33_33 //👇🏻 -- Sends the swapped image to the user--_33 await io.resend.sendEmail("send-email", {_33 from: "[email protected]",_33 to: [email],_33 subject: "Your avatar is ready! 🌟🤩",_33 text: `Hi! \n View and download your avatar here - ${swappedImage.output}`,_33 });_33_33 await io.logger.info(_33 "✨ Congratulations, the image has been delivered! ✨"_33 );_33 },_33});
Learn more
For a full overview of how to make this project yourself, check out our blog post.